能不能用Java写一个简单的飞机大战小游戏的代码
时间: 2024-01-29 16:03:15 浏览: 29
当然可以!下面是一个简单的Java飞机大战小游戏的代码示例:
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class PlaneGame extends JFrame implements ActionListener, Runnable {
private static final long serialVersionUID = 1L;
// 定义面板和按钮
JPanel panel = new JPanel();
JButton startButton = new JButton("开始游戏");
JButton endButton = new JButton("结束游戏");
MyPlane myPlane = null;
public PlaneGame() {
// 设置窗口大小和标题
setSize(400, 600);
setTitle("飞机大战");
// 设置面板布局和添加面板组件
panel.setLayout(new FlowLayout());
panel.add(startButton);
panel.add(endButton);
// 添加面板和设置监听器
add(panel, BorderLayout.NORTH);
startButton.addActionListener(this);
endButton.addActionListener(this);
// 设置窗口可见
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public void actionPerformed(ActionEvent e) {
// 处理按钮事件
if (e.getSource() == startButton) {
start();
} else if (e.getSource() == endButton) {
end();
}
}
public void start() {
// 开始游戏
myPlane = new MyPlane(this);
Thread t = new Thread(myPlane);
t.start();
}
public void end() {
// 结束游戏
myPlane.stop();
}
public static void main(String[] args) {
new PlaneGame();
}
public void paint(Graphics g) {
// 画出游戏界面
super.paint(g);
g.setColor(Color.BLUE);
g.fillRect(0, 0, 400, 600);
}
@Override
public void run() {
// TODO Auto-generated method stub
}
}
class MyPlane implements Runnable {
PlaneGame game = null;
int x = 180;
int y = 500;
boolean flag = true;
public MyPlane(PlaneGame game) {
this.game = game;
}
public void draw(Graphics g) {
// 画出飞机
g.setColor(Color.RED);
g.fillRect(x, y, 40, 40);
}
public void move() {
// 移动飞机
if (flag) {
x = x + 5;
} else {
x = x - 5;
}
if (x > 360 || x < 0) {
flag = !flag;
}
}
public void stop() {
// 结束游戏
flag = false;
}
@Override
public void run() {
// 不断重绘和移动飞机
while (flag) {
game.repaint();
move();
try {
Thread.sleep(50);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
```
上面的代码实现了一个简单的飞机大战小游戏,包括开始和结束游戏按钮、飞机的移动和绘制等功能。你可以根据自己的需求进行修改和扩展。
相关推荐
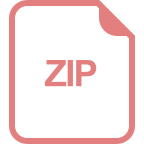
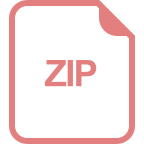














