选择合适的结构表示图,在此基础上实现拓扑排序算法。 要求:对所设计的图结构,提供必要的基本功能
时间: 2023-12-14 11:36:13 浏览: 30
以下是一个基本的图结构及其相关的拓扑排序算法实现:
```python
class Graph:
def __init__(self):
self.vertices = {}
self.indegree = {}
def add_vertex(self, vertex):
if vertex not in self.vertices:
self.vertices[vertex] = []
self.indegree[vertex] = 0
def add_edge(self, u, v):
if u not in self.vertices:
self.add_vertex(u)
if v not in self.vertices:
self.add_vertex(v)
self.vertices[u].append(v)
self.indegree[v] += 1
def topological_sort(self):
queue = []
for vertex in self.vertices:
if self.indegree[vertex] == 0:
queue.append(vertex)
sorted_vertices = []
while queue:
vertex = queue.pop(0)
sorted_vertices.append(vertex)
for adjacent_vertex in self.vertices[vertex]:
self.indegree[adjacent_vertex] -= 1
if self.indegree[adjacent_vertex] == 0:
queue.append(adjacent_vertex)
if len(sorted_vertices) != len(self.vertices):
raise Exception("Graph has at least one cycle")
return sorted_vertices
```
这里使用了字典来表示图结构,其中键表示每个节点,值是一个列表,表示该节点连接的其他节点。通过一个字典记录每个节点的入度,以便在拓扑排序过程中判断是否已经处理完该节点的所有入边。
拓扑排序算法的实现使用了队列,从入度为 0 的节点开始进行处理,将其加入排序结果中,然后将该节点连接的所有节点的入度减 1,如果减 1 后入度为 0,则将其加入队列中。依次类推,直到队列为空,此时所有节点都已经处理完成,如果排序结果中的节点数量不等于图中节点的数量,则说明图中存在环。
相关推荐
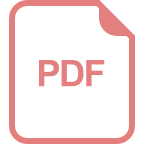
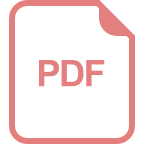
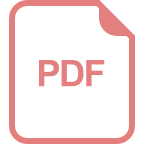














