用虚幻引擎C++插件 写一个虚幻引擎安卓项目调用 安卓原生的 WebView 展示网页,给出具体详细的实现代码
时间: 2024-03-30 13:33:18 浏览: 18
好的,以下是基于 Unreal Engine 4.26 的 C++ 插件项目示例代码,用于在 Android 平台上调用 Android 原生 WebView 并加载网页:
首先,在您的插件项目中,您需要创建一个名为“WebViewPlugin”的 C++ 类。该类将包含所有与 WebView 相关的代码。以下是 WebViewPlugin.h 文件的示例代码:
```cpp
#pragma once
#include "CoreMinimal.h"
#include "Modules/ModuleManager.h"
class FWebViewPluginModule : public IModuleInterface
{
public:
static inline FWebViewPluginModule& Get()
{
return FModuleManager::LoadModuleChecked<FWebViewPluginModule>("WebViewPlugin");
}
static inline bool IsAvailable()
{
return FModuleManager::Get().IsModuleLoaded("WebViewPlugin");
}
virtual void StartupModule() override;
virtual void ShutdownModule() override;
};
```
接下来,在 WebViewPlugin.cpp 文件中,您需要实现 StartupModule() 和 ShutdownModule() 函数,并在 StartupModule() 函数中初始化 WebView。以下是示例代码:
```cpp
#include "WebViewPlugin.h"
#include "Modules/ModuleManager.h"
#include "PlatformWebView.h"
void FWebViewPluginModule::StartupModule()
{
TSharedPtr<IPlatformWebView> PlatformWebView = FPlatformWebViewModule::Get().CreatePlatformWebView();
if (PlatformWebView.IsValid())
{
PlatformWebView->Initialize();
PlatformWebView->LoadURL("https://www.example.com");
}
}
void FWebViewPluginModule::ShutdownModule()
{
TSharedPtr<IPlatformWebView> PlatformWebView = FPlatformWebViewModule::Get().GetPlatformWebView();
if (PlatformWebView.IsValid())
{
PlatformWebView->CloseWindow();
}
}
IMPLEMENT_MODULE(FWebViewPluginModule, WebViewPlugin)
```
在上面的示例代码中,我们使用 FPlatformWebViewModule 类来创建和初始化 WebView。该类是一个抽象类,它定义了与平台无关的接口,您可以使用它来访问特定平台的 WebView。
在 Android 平台上,您需要实现 IPlatformWebView 接口,并使用 JNI 调用 Android 原生 WebView。以下是 PlatformWebViewAndroid.h 和 PlatformWebViewAndroid.cpp 文件的示例代码:
PlatformWebViewAndroid.h:
```cpp
#pragma once
#include "CoreMinimal.h"
#include "PlatformWebView.h"
class FPlatformWebViewAndroid : public IPlatformWebView
{
public:
virtual void Initialize() override;
virtual void LoadURL(const FString& URL) override;
virtual void CloseWindow() override;
};
```
PlatformWebViewAndroid.cpp:
```cpp
#include "PlatformWebViewAndroid.h"
#include "Android/AndroidApplication.h"
#include "Android/AndroidJNI.h"
#include "Android/AndroidJava.h"
void FPlatformWebViewAndroid::Initialize()
{
JNIEnv* Env = FAndroidApplication::GetJavaEnv();
if (Env)
{
FJavaClassObject WebViewClass(Env, FJavaWrapper::GameActivityClassID, FJavaWrapper::GameActivityThis);
if (WebViewClass)
{
FJavaObjectMethod Method(Env, WebViewClass, "initializeWebView", "()V");
if (Method)
{
Method.Call();
}
}
}
}
void FPlatformWebViewAndroid::LoadURL(const FString& URL)
{
JNIEnv* Env = FAndroidApplication::GetJavaEnv();
if (Env)
{
jstring JURL = Env->NewStringUTF(TCHAR_TO_UTF8(*URL));
if (JURL)
{
FJavaClassObject WebViewClass(Env, FJavaWrapper::GameActivityClassID, FJavaWrapper::GameActivityThis);
if (WebViewClass)
{
FJavaObjectMethod Method(Env, WebViewClass, "loadURL", "(Ljava/lang/String;)V");
if (Method)
{
Method.Call(JURL);
}
}
Env->DeleteLocalRef(JURL);
}
}
}
void FPlatformWebViewAndroid::CloseWindow()
{
JNIEnv* Env = FAndroidApplication::GetJavaEnv();
if (Env)
{
FJavaClassObject WebViewClass(Env, FJavaWrapper::GameActivityClassID, FJavaWrapper::GameActivityThis);
if (WebViewClass)
{
FJavaObjectMethod Method(Env, WebViewClass, "closeWebView", "()V");
if (Method)
{
Method.Call();
}
}
}
}
```
在上面的示例代码中,我们使用 FJavaClassObject 和 FJavaObjectMethod 类来调用 Android 原生 WebView。这些类是 Unreal Engine 中的封装类,它们可以简化 JNI 调用。
最后,在您的插件项目中,您需要添加 AndroidManifest.xml 文件,并在其中添加必要的权限和配置项。以下是示例代码:
```xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.YourCompany.WebViewPlugin"
android:versionCode="1"
android:versionName="1.0" >
<uses-permission android:name="android.permission.INTERNET" />
<uses-feature android:name="android.hardware.touchscreen" android:required="false" />
<application android:label="@string/app_name" android:icon="@drawable/icon">
<activity android:name="com.epicgames.ue4.GameActivity"
android:label="@string/app_name"
android:launchMode="singleTask"
android:screenOrientation="landscape"
android:configChanges="keyboardHidden|orientation|screenSize">
<meta-data android:name="android.app.lib_name" android:value="WebViewPlugin" />
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
```
在上面的示例代码中,我们添加了 INTERNET 权限和一个活动标记,以便在 AndroidManifest.xml 文件中注册插件活动。
希望这个示例代码能够帮助您实现在 Unreal Engine 4 中调用 Android 原生 WebView 并加载网页的功能。
相关推荐
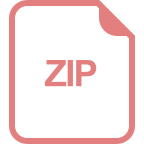
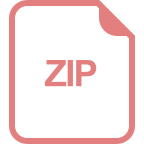
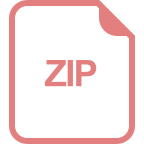













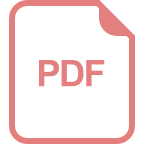