基于循环队列的银行排队叫号系统c++要求包含取号进队、排队等候、叫号服务、当前不排队,余下依次等待、下班
时间: 2023-05-28 15:07:10 浏览: 101
#include <stdio.h>
#include <stdlib.h>
#define MAX_QUEUE_SIZE 10 // 队列的最大长度
typedef struct {
int num; // 号码
int isServed; // 是否已经服务
} Customer;
typedef struct {
Customer data[MAX_QUEUE_SIZE]; // 队列元素数组
int front; // 队列头指针
int rear; // 队列尾指针
} Queue;
void initQueue(Queue *q) { // 初始化队列
q->front = q->rear = 0;
}
int isQueueEmpty(Queue *q) { // 判断队列是否为空
return q->front == q->rear;
}
int isQueueFull(Queue *q) { // 判断队列是否为满
return (q->rear + 1) % MAX_QUEUE_SIZE == q->front;
}
void enQueue(Queue *q, Customer item) { // 入队
if (isQueueFull(q)) {
printf("队列已满,无法继续排队!\n");
return;
}
q->data[q->rear] = item;
q->rear = (q->rear + 1) % MAX_QUEUE_SIZE;
}
Customer deQueue(Queue *q) { // 出队
if (isQueueEmpty(q)) {
printf("队列为空,无法叫号!\n");
return (Customer){0, 0};
}
Customer item = q->data[q->front];
q->front = (q->front + 1) % MAX_QUEUE_SIZE;
return item;
}
void printQueue(Queue *q) { // 输出队列中的所有元素
printf("当前排队的顾客有:\n");
int i = q->front;
while (i != q->rear) {
printf("%d ", q->data[i].num);
i = (i + 1) % MAX_QUEUE_SIZE;
}
printf("\n");
}
void serveCustomer(Customer *c) { // 服务一个顾客
printf("请%d号顾客到窗口服务!\n", c->num);
c->isServed = 1;
}
int main() {
Queue q;
initQueue(&q);
int num = 0;
while (1) { // 不断地读入用户的操作并执行
printf("请输入您要进行的操作:(1.取号进队 2.排队等候 3.叫号服务 4.当前不排队 5.下班)\n");
int choice;
scanf("%d", &choice);
if (choice == 1) { // 取号进队
if (isQueueFull(&q)) {
printf("队列已满,无法继续排队!\n");
} else {
num++;
Customer c = {num, 0};
enQueue(&q, c);
printf("您的号码为%d,请耐心等待!\n", num);
}
} else if (choice == 2) { // 排队等候
printQueue(&q);
} else if (choice == 3) { // 叫号服务
Customer c = deQueue(&q);
if (c.isServed) {
printf("该顾客已经被服务过了!\n");
} else {
serveCustomer(&c);
}
} else if (choice == 4) { // 当前不排队
printf("您已经退出排队!\n");
} else if (choice == 5) { // 下班
printf("今天的工作已经结束,下班!\n");
break;
} else { // 输入不合法
printf("输入不合法,请重新输入!\n");
}
}
return 0;
}
阅读全文
相关推荐

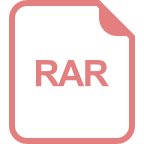
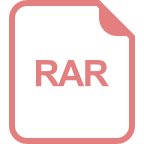
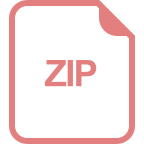
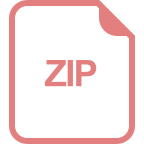
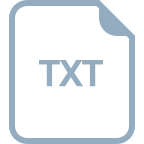
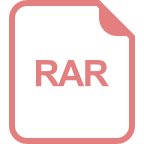
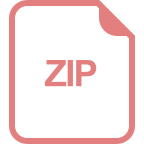
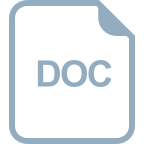
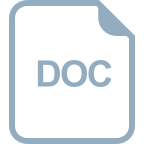
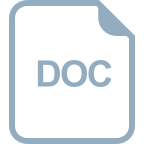
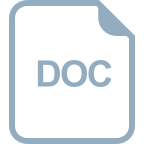


