写一个银行排队叫号系统c++
时间: 2024-12-14 16:21:17 浏览: 17
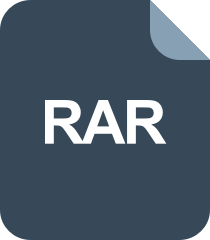
银行排队叫号系统

一个简单的银行排队叫号系统可以利用C++的基本结构设计。这里提供一个基本框架,使用线程模拟客户、柜员以及管理队列的过程:
```cpp
#include <iostream>
#include <thread>
#include <queue>
#include <mutex>
// 客户类
class Customer {
public:
int id;
void checkIn();
};
void Customer::checkIn() {
std::cout << "Customer " << id << " checking in." << std::endl;
std::unique_lock<std::mutex> lock(queue_mutex);
queue.push(this);
// 模拟处理时间
std::this_thread::sleep_for(std::chrono::seconds(5));
std::cout << "Customer " << id << " being served by counter " << current_counter << "." << std::endl;
}
// 柜员类,用于服务客户
class Teller {
private:
static std::queue<Customer*> queue;
static std::mutex queue_mutex;
static int current_counter;
public:
static void serveNext();
};
std::queue<Customer*> Teller::queue;
std::mutex Teller::queue_mutex;
int Teller::current_counter = 0;
void Teller::serveNext() {
if (not Teller::queue.empty()) {
Customer* customer = Teller::queue.front();
Teller::queue.pop();
current_counter++;
customer->checkIn();
} else {
std::cout << "No customers waiting." << std::endl;
}
}
int main() {
for (int i = 1; i <= 10; ++i) { // 创建10位客户
std::thread customer_thread(Customer{i}, &Teller::serveNext);
customer_thread.detach(); // 启动客户线程并立即返回主线程
}
while (true) {
Teller::serveNext(); // 主线程不断检查是否有新任务
}
return 0;
}
```
在这个例子中,`Customer`类代表需要服务的客户,`Teller`类模拟柜员。每个客户线程会自动加入队列等待,然后柜员线程会循环处理队列中的客户。注意这只是一个基础模型,实际应用可能会包含更复杂的功能如叫号显示、客户状态跟踪等。
阅读全文
相关推荐
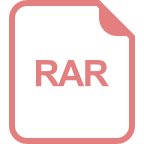
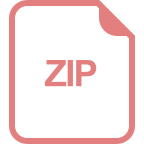
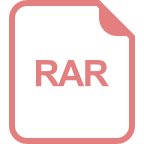


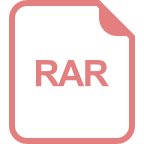
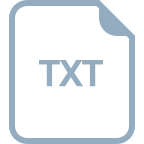
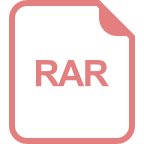
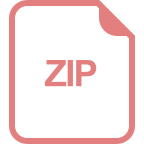
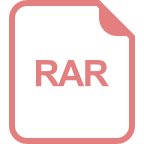
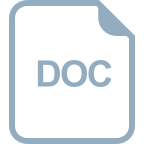
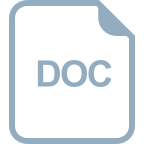
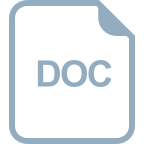



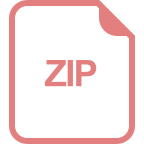