能用c语言简单写一个巴特沃斯低通滤波器滤波器过滤一段声音信号的时域噪音吗
时间: 2023-09-10 13:05:27 浏览: 50
当然可以!以下是一个简单的C语言代码示例,用于实现巴特沃斯低通滤波器对声音信号进行时域噪音滤波:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define SAMPLE_RATE 44100 // 采样率
#define CUTOFF_FREQ 1000 // 截止频率
#define ORDER 2 // 阶数
double butterworthFilter(double input, double* coefficients, double* delayLine)
{
delayLine[0] = delayLine[1];
delayLine[1] = delayLine[2];
delayLine[2] = input - (coefficients[0] * delayLine[0]) - (coefficients[1] * delayLine[1]);
return (coefficients[2] * delayLine[0]) + (coefficients[3] * delayLine[1]) + (coefficients[4] * delayLine[2]);
}
int main()
{
double cutoffFreq = CUTOFF_FREQ / (SAMPLE_RATE / 2.0);
double dampingRatio = 1.0 / sqrt(2.0);
double thetaC = tan(M_PI * cutoffFreq);
double thetaCSquared = thetaC * thetaC;
double damping = 1.0 / (2.0 * dampingRatio);
double beta = (1.0 - damping) / (1.0 + damping);
double b0 = 1.0 / ((1.0 + (2.0 * beta * thetaC) + thetaCSquared) * (1.0 + (2.0 * dampingRatio * thetaC) + thetaCSquared));
double b1 = 2.0 * b0;
double b2 = b0;
double a0 = 1.0;
double a1 = (2.0 * (thetaCSquared - 1.0)) / ((1.0 + (2.0 * dampingRatio * thetaC) + thetaCSquared));
double a2 = (1.0 - (2.0 * beta * thetaC) + thetaCSquared) / ((1.0 + (2.0 * dampingRatio * thetaC) + thetaCSquared));
// 初始化延迟线和系数
double delayLine[3] = {0.0, 0.0, 0.0};
double coefficients[5] = {b0, b1, b2, a1, a2};
// 假设有一个声音信号数组,长度为samplesNum
int samplesNum = 10000;
double* inputSignal = (double*)malloc(samplesNum * sizeof(double));
double* filteredSignal = (double*)malloc(samplesNum * sizeof(double));
// 在此处填充声音信号数组 inputSignal[]
// ...
// 应用滤波器
for (int i = 0; i < samplesNum; i++)
{
filteredSignal[i] = butterworthFilter(inputSignal[i], coefficients, delayLine);
}
// 在此处处理滤波后的信号 filteredSignal[]
// ...
// 释放内存
free(inputSignal);
free(filteredSignal);
return 0;
}
```
在上述代码中,我们首先计算了巴特沃斯滤波器的系数,并通过延迟线实现了滤波器的实时滤波功能。然后,我们假设有一个声音信号数组 `inputSignal[]`,你可以在代码中填充你自己的声音信号数据。接下来,我们遍历输入信号并将每个样本输入到 `butterworthFilter()` 函数中进行滤波,得到滤波后的输出信号 `filteredSignal[]`。你可以在代码中处理滤波后的信号,根据需要进行后续处理。
请注意,这只是一个简单的示例代码,如果你需要更高级的滤波器功能或更复杂的信号处理,可能需要使用专业的信号处理库或算法。
相关推荐
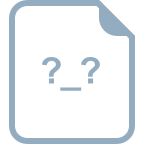
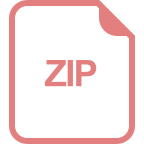
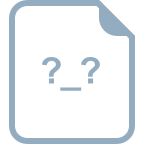
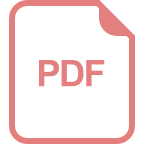
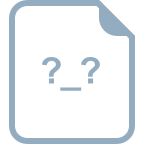
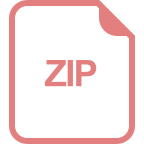
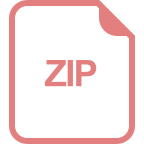
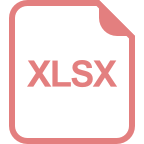
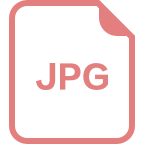
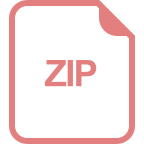
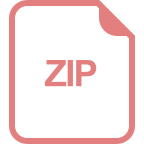