编写一个程序,统计一段文本中每个单词出现的次数,并将结果存储在字典中 Python
时间: 2024-12-13 18:22:41 浏览: 12
在Python中,你可以使用`collections`模块的`Counter`类来轻松完成这个任务。下面是一个简单的程序,它可以接受一段文本作为输入,然后统计每个单词的频率:
```python
from collections import Counter
def word_count(text):
# 清理文本,移除标点符号并将所有字母转为小写
words = text.lower().replace(",", "").replace(".", "").split()
# 使用Counter计算每个单词出现的次数
word_counts = Counter(words)
# 返回包含词频的字典
return dict(word_counts)
# 测试文本
text = "This is a sample text. It contains some words like this, which will be counted. Counting is fun!"
word_freq_dict = word_count(text)
print(word_freq_dict)
相关问题
编写一个程序,要求用户输入一段英文文本,统计文本中每个单词出现的次数,并输出统计结果。
这是一个常见的文本分析任务,可以使用Python编写一个简单的程序来完成。以下是使用Python的解决方案:
```python
def word_count(text):
# 将文本转换为小写并分割成单词列表
words = text.lower().split()
# 创建一个空字典来存储单词及其计数
word_dict = {}
# 遍历单词列表,更新字典中的计数
for word in words:
if word in word_dict:
word_dict[word] += 1
else:
word_dict[word] = 1
return word_dict
# 获取用户输入的英文文本
input_text = input("请输入一段英文文本:")
# 调用函数并打印结果
result = word_count(input_text)
for word, count in result.items():
print(f"{word}: {count}")
编写一个程序,要求用户输入一段英文文本,统计文本中每个单词出现的次数,并输出统计
编写一个简单的Python程序来实现这个功能:
```python
def word_count(text):
# 将文本转换为小写并分割成单词列表
words = text.lower().split()
# 创建一个空字典存储单词及其计数
word_dict = {}
# 遍历单词列表,更新字典中的计数
for word in words:
if word in word_dict:
word_dict[word] += 1
else:
word_dict[word] = 1
return word_dict
# 请求用户输入文本
user_text = input("请输入一段英文文本: ")
# 统计并打印结果
word_counts = word_count(user_text)
for word, count in word_counts.items():
print(f"{word}: {count} 次")
阅读全文
相关推荐
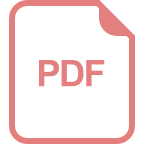
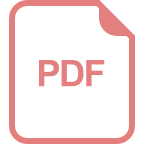
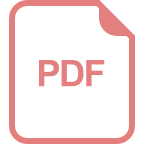













