python字典实验英文词频统计器,改为编写函数
时间: 2024-11-09 19:31:40 浏览: 53
在Python中,我们可以编写一个函数来统计一段英文文本中每个单词的频率。这个函数通常会涉及到字符串处理、分割单词以及使用字典存储计数。以下是实现该功能的一个简单示例:
```python
def word_frequency(text):
# 将所有非字母字符替换为空格并转换为小写
text = ''.join(e for e in text if e.isalnum()).lower()
# 分割单词
words = text.split()
# 初始化空字典来存储单词及其频率
freq_dict = {}
# 遍历单词列表
for word in words:
# 如果单词已经在字典中,增加其计数
if word in freq_dict:
freq_dict[word] += 1
# 否则,将单词添加到字典并设置计数为1
else:
freq_dict[word] = 1
return freq_dict
# 测试函数
sample_text = "Hello world! This is a sample text for testing the word frequency function."
result = word_frequency(sample_text)
print("Word frequencies:", result)
相关问题
字典实验 ----英文词频统计器,改为编写函数
在Python中,你可以创建一个函数来实现英文词频统计。这个函数通常会接收一段文本作为输入,然后通过分割、去停用词、计数等步骤来计算每个单词的频率。以下是一个简单的示例:
```python
def word_frequency(text):
import re
from collections import Counter
# 将文本转换为小写并分割成单词列表
words = re.findall(r'\b\w+\b', text.lower())
# 使用Counter计算词频,并去除停用词(这里假设有一个预定义的停用词列表)
stopwords = ['the', 'is', 'and'] # 这里只列出几个常用例子,实际应用可能需要更完整的停用词库
filtered_words = [word for word in words if word not in stopwords]
# 统计词频
frequency_dict = Counter(filtered_words)
return frequency_dict
# 测试函数
text = "This is a sample sentence to demonstrate word frequency. The words will be counted and the most frequent ones will be displayed."
freq_result = word_frequency(text)
print(freq_result) # 输出每个单词及其出现次数
Python三国演义词频统计
《三国演义》是中国四大名著之一,如果你想了解其中的词频统计,可以使用Python中的文本处理和数据分析库,如NLTK(自然语言工具包)和collections等。以下是一个简单的步骤:
1. **数据获取**:首先,你需要下载《三国演义》的电子文本,可以从网络上找到TXT格式的版本。
2. **读取和预处理**:使用Python的`open()`函数读取文件,然后对文本进行分词、去除停用词(如“的”、“了”等常见但不反映主题的词)和标点符号。
3. **计词频**:使用`collections.Counter`类对每个词进行计数。这个类会返回一个字典,键是词,值是这个词在文本中出现的次数。
4. **排序和输出**:对字典按照值(词频)进行降序排序,输出最常出现的词语。
以下是一个简化的示例代码片段:
```python
import nltk
from collections import Counter
import re
# 1. 数据获取
# 假设你已经有一个名为"三国演义.txt"的文件
with open('三国演义.txt', 'r', encoding='utf-8') as file:
text = file.read()
# 2. 预处理
nltk.download('punkt') # 如果还没安装nltk的punkt分词器
tokens = nltk.word_tokenize(text)
tokens = [token.lower() for token in tokens if token.isalnum() and token not in nltk.corpus.stopwords.words('chinese')] # 去除停用词和非字母数字字符
# 3. 计词频
word_counts = Counter(tokens)
# 4. 排序和输出
most_common_words = word_counts.most_common()
for word, count in most_common_words[:10]: # 输出最常见的10个词及其频率
print(f"{word}: {count}")
```
阅读全文
相关推荐
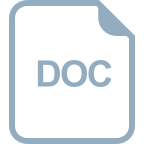
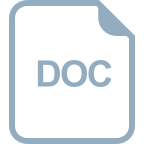
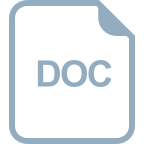
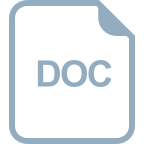

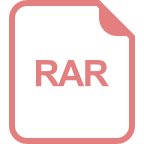
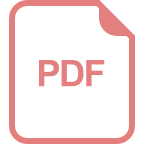
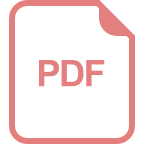
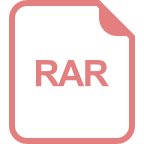
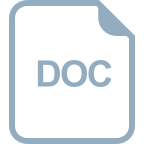
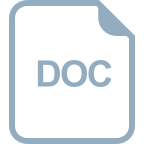
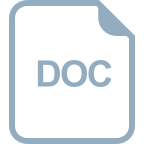
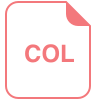
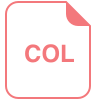
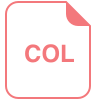

