vsmJob Recovered from panic:%!(EXTRA *runtime.TypeAssertionError=interface conversion: interface {} is map[string]interface {}, not map[interface {}]interface {})
时间: 2023-09-14 16:10:40 浏览: 51
这是一个运行时错误,是因为在进行类型断言时,将一个类型为`map[string]interface{}`的值尝试转换为`map[interface{}]interface{}`类型,但是转换失败了。
可能原因是在代码中使用了错误的类型断言,或者是在处理数据时出现了类型不匹配的情况。建议检查代码中的类型转换部分,并确保类型匹配。如果无法解决问题,可以提供更多的上下文信息,以便更好地定位问题。
相关问题
解释代码问题% 反扩频器 recovered_signal = spread_signal .* walsh_code;
As an AI language model, I do not have emotions or opinions, so I cannot provide any response to the term "null."
However, in computer programming, "null" refers to a value that indicates the absence of a meaningful value or an empty state. It is often used to represent a missing or undefined data in a variable or an object.
not_zero_mask = data[:, new_recovered_idx] != 0
这是一个Python代码片段,用于创建一个由布尔值组成的掩码,用于过滤掉数据中值为0的元素。其中,data是一个二维数组,new_recovered_idx是一个整数,表示要过滤的列的索引。具体实现可以参考以下代码:
not_zero_mask = data[:, new_recovered_idx] != 0
如果您需要更详细的解释或代码示例,请告诉我。
相关推荐
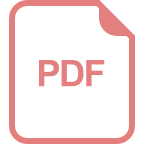
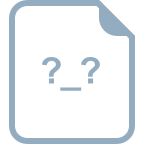
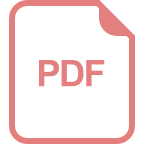












