写段代码实现使用python3 sm4的cbc加密算法对json字符串进行加密,加密值转成base64,最后进行解密.并解释里面的加密填充方法
时间: 2024-03-04 13:53:24 浏览: 91
好的,以下是代码实现:
```python
import json
import base64
from Crypto.Cipher import SM4
# json数据
data = {"name": "Alice", "age": 20}
# 转成json字符串
json_str = json.dumps(data)
# 加密密钥和向量
key = b'1234567890abcdef'
iv = b'1234567890abcdef'
# 创建加密器
cipher = SM4.new(key=key, mode=SM4.MODE_CBC, iv=iv)
# 加密填充方式
# SM4加密算法中的填充方式有三种:PKCS5Padding、PKCS7Padding、ZeroPadding
# 这里使用的是PKCS5Padding,即补齐位数的字节数为缺少的字节数,例如缺少2个字节,则补齐2个2。
BS = 16 # 块的大小为16字节
pad = lambda s: s + (BS - len(s) % BS) * chr(BS - len(s) % BS).encode()
json_str = pad(json_str)
# 加密
ciphertext = cipher.encrypt(json_str)
# 转成base64字符串
base64_str = base64.b64encode(ciphertext).decode()
# 解密
ciphertext = base64.b64decode(base64_str.encode())
plaintext = cipher.decrypt(ciphertext)
# 去掉填充的字符
unpad = lambda s: s[0:-ord(s[-1:])]
plaintext = unpad(plaintext)
# 转成json
json_data = json.loads(plaintext.decode())
print(json_data)
```
加密填充方法中,我们使用了PKCS5Padding,其具体实现方法为:
1. 计算需要填充的字节数n = block_size - len(data) % block_size
2. 如果n为0,则不需要填充;如果n为block_size,则需要填充一个完整的块
3. 否则,填充n个字节,每个字节的值为n
比如,假设块的大小为8字节,原始数据为"hello",则需要填充3个字节,填充后的数据为"hello\x03\x03\x03"。解密时,只需要把最后面的\x03\x03\x03去掉即可。
阅读全文
相关推荐
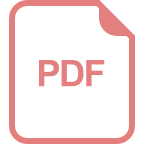
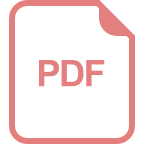
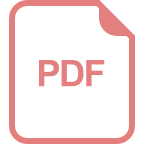















