使用c++写个使用boost库中函数 获取当前时间
时间: 2023-12-10 09:42:18 浏览: 29
可以使用Boost库中的posix_time模块来获取当前时间。下面是一个使用Boost库获取当前时间的示例代码:
```c++
#include <boost/date_time/posix_time/posix_time.hpp>
#include <iostream>
int main()
{
// 获取当前时间
boost::posix_time::ptime now = boost::posix_time::microsec_clock::local_time();
// 格式化输出时间
std::string str_time = boost::posix_time::to_simple_string(now);
std::cout << "Current time: " << str_time << std::endl;
return 0;
}
```
输出的结果类似于:
```
Current time: 2021-Aug-13 22:59:59.999999
```
可以看到,获取当前时间需要使用`boost::posix_time::microsec_clock::local_time()`函数,该函数返回的是一个`boost::posix_time::ptime`类型的时间戳。然后,可以使用`boost::posix_time::to_simple_string()`函数将时间戳格式化为字符串。
相关问题
c++ boost库常用函数
Boost库是一个功能强大的C++库集合,提供了许多常用的函数和模块。以下是一些常用的Boost库函数:
1. 字符串和文本处理库:Boost库提供了丰富的字符串处理功能,比如lexical_cast用于数值转换,format用于字符串格式化,string_algo用于字符串算法。
2. 类型推导:Boost库提供了BOOST_AUTO和BOOST_TYPEOF用于类型推导,可以方便地获取表达式的类型。
3. 智能指针:Boost库提供了多种智能指针,如scoped_ptr、shared_ptr、weak_ptr等,用于管理动态分配的内存。
4. 数组和容器:Boost库提供了多维数组multi_array、动态多维数组、普通数组array、散列容器unordered_set、unordered_map、双向映射容器bimap、环形缓冲区circular_buffer等,方便了数组和容器的操作和管理。
5. XML和JSON解析:Boost库提供了property_tree模块,可用于解析和处理XML和JSON数据。
6. 简化循环:Boost库提供了BOOST_FOREACH宏,可用于简化循环操作。
7. 随机数库:Boost库提供了Random模块,用于生成随机数。
8. 引用库:Boost库提供了ref模块,用于处理引用。
9. 绑定库:Boost库提供了bind模块,用于函数对象的绑定。
10. 线程库:Boost库提供了thread模块,用于跨平台多线程编程。
这些只是Boost库的一部分常用函数,Boost库还有许多其他功能和模块可供使用。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [C++ Boost库:工厂函数 make_shared](https://blog.csdn.net/u014779536/article/details/116400788)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT0_1"}}] [.reference_item style="max-width: 50%"]
- *2* *3* [【BOOST C++库】BOOST C++库20个分类指南](https://blog.csdn.net/gongdiwudu/article/details/127961836)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT0_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
c++ boost库使用
Boost是一个为C++开发者提供的一组高质量、可重用的程序库,包括诸如日期时间、正则表达式、文件系统、智能指针、网络、线程等方面的库。Boost库的使用可以帮助C++开发者轻松地实现一些常见的功能,提高代码的复用性和可维护性。
下面是使用Boost库的步骤:
1. 下载并安装Boost库:从Boost官网下载最新版本的Boost库,并按照官方文档中的说明进行安装。
2. 引入头文件:在C++源文件中使用Boost库时,需要在代码中引入相应的头文件,例如:
```c++
#include <boost/regex.hpp> // 正则表达式库
#include <boost/filesystem.hpp> // 文件系统库
#include <boost/thread.hpp> // 线程库
// 其他库的头文件
```
3. 编写代码:根据需要使用Boost库提供的函数和类,编写相应的代码实现所需功能。
4. 编译链接:使用C++编译器编译代码时,需要将Boost库的头文件路径和库文件路径添加到编译选项中,并将所需的Boost库文件链接到可执行文件中。
例如,在Linux系统下使用g++编译器编译代码时,可以使用以下命令:
```
g++ -I /usr/local/boost/include -L /usr/local/boost/lib -lboost_regex -lboost_filesystem -lboost_thread -o program program.cpp
```
其中,-I选项指定Boost库的头文件路径,-L选项指定Boost库的库文件路径,-l选项指定需要链接的Boost库文件名。
5. 运行程序:将编译生成的可执行文件运行即可。
相关推荐
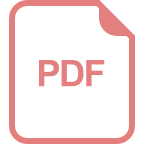
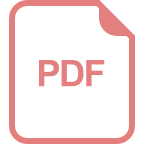
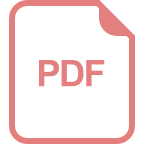












