Java swing 点击按钮复制文件到当前目录
时间: 2023-09-12 07:07:53 浏览: 54
您可以使用Java的Swing库中的JFileChooser组件来让用户选择要复制的文件,然后使用Java IO库中的FileInputStream和FileOutputStream来进行文件复制操作。
以下是一个示例代码:
```java
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
public class FileCopyExample {
public static void main(String[] args) {
JFrame frame = new JFrame("File Copy Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel();
JButton copyButton = new JButton("Copy File");
copyButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
JFileChooser fileChooser = new JFileChooser();
int result = fileChooser.showOpenDialog(frame);
if (result == JFileChooser.APPROVE_OPTION) {
File selectedFile = fileChooser.getSelectedFile();
String destinationFilePath = selectedFile.getName() + "_copy";
File destinationFile = new File(destinationFilePath);
try {
FileInputStream inputStream = new FileInputStream(selectedFile);
FileOutputStream outputStream = new FileOutputStream(destinationFile);
byte[] buffer = new byte[1024];
int length;
while ((length = inputStream.read(buffer)) > 0) {
outputStream.write(buffer, 0, length);
}
inputStream.close();
outputStream.close();
JOptionPane.showMessageDialog(panel, "File copied successfully!");
} catch (Exception ex) {
ex.printStackTrace();
JOptionPane.showMessageDialog(panel, "Error copying file!");
}
}
}
});
panel.add(copyButton);
frame.add(panel);
frame.pack();
frame.setVisible(true);
}
}
```
这个程序创建了一个简单的Swing窗口,其中包含一个按钮。单击按钮时,程序会打开文件选择对话框,让用户选择要复制的文件。然后,程序会将选定的文件复制到当前目录下并添加“_copy”后缀。在复制完成后,程序会弹出一个消息框提示用户操作成功或失败。
相关推荐
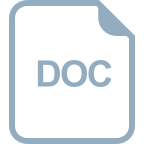
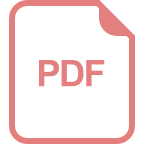
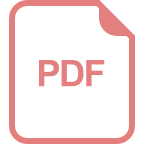









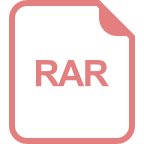
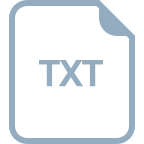
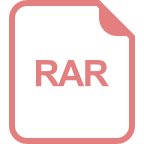
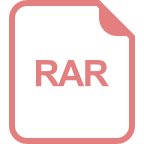
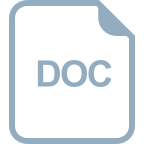
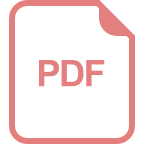