用Java写一个交通旅游图的最短路径问题
时间: 2023-10-07 14:05:06 浏览: 95
好的,我可以为您提供一个使用Java语言实现的交通旅游图最短路径算法,以下是实现的步骤:
1. 首先,我们需要定义一个图类和一个节点类。节点类需要包含节点的名称、与其他节点相邻的边和边的权重信息。图类需要包含节点的集合和与节点相邻的边的集合。
2. 然后,我们需要实现一个Dijkstra算法来求解最短路径。Dijkstra算法的基本思想是从起点开始,每次选择距离最近的一个节点,然后更新与其相邻的节点的距离。直到更新完所有节点,就得到了最短路径。
3. 接下来,我们可以使用优先队列来实现Dijkstra算法。每次从队列中选择距离最短的节点,然后更新其相邻节点的距离。这个过程可以使用一个数组来保存每个节点的最短距离和路径信息。
4. 最后,我们可以根据起点和终点节点,从数组中回溯得到最短路径信息。
以下是Java代码实现的示例:
```java
import java.util.*;
public class Graph {
private final Map<String, Node> nodes = new HashMap<>();
public void addNode(String name) {
nodes.putIfAbsent(name, new Node(name));
}
public void addEdge(String sourceName, String destName, int weight) {
Node source = nodes.get(sourceName);
Node dest = nodes.get(destName);
source.addEdge(new Edge(dest, weight));
}
public void dijkstra(String startName) {
Node start = nodes.get(startName);
PriorityQueue<Node> queue = new PriorityQueue<>();
start.setDistance(0);
queue.offer(start);
while (!queue.isEmpty()) {
Node curr = queue.poll();
for (Edge edge : curr.getEdges()) {
Node next = edge.getDest();
int newDistance = curr.getDistance() + edge.getWeight();
if (newDistance < next.getDistance()) {
queue.remove(next);
next.setDistance(newDistance);
next.setPath(new LinkedList<>(curr.getPath()));
next.getPath().add(curr);
queue.offer(next);
}
}
}
}
public List<Node> getShortestPath(String endName) {
return nodes.get(endName).getPath();
}
private static class Node implements Comparable<Node> {
private final String name;
private final List<Edge> edges = new ArrayList<>();
private int distance = Integer.MAX_VALUE;
private List<Node> path = new LinkedList<>();
public Node(String name) {
this.name = name;
}
public void addEdge(Edge edge) {
edges.add(edge);
}
public List<Edge> getEdges() {
return edges;
}
public int getDistance() {
return distance;
}
public void setDistance(int distance) {
this.distance = distance;
}
public List<Node> getPath() {
return path;
}
public void setPath(List<Node> path) {
this.path = path;
}
@Override
public int compareTo(Node other) {
return Integer.compare(distance, other.distance);
}
}
private static class Edge {
private final Node dest;
private final int weight;
public Edge(Node dest, int weight) {
this.dest = dest;
this.weight = weight;
}
public Node getDest() {
return dest;
}
public int getWeight() {
return weight;
}
}
}
```
使用示例:
```java
public class Main {
public static void main(String[] args) {
Graph graph = new Graph();
graph.addNode("A");
graph.addNode("B");
graph.addNode("C");
graph.addNode("D");
graph.addNode("E");
graph.addEdge("A", "B", 2);
graph.addEdge("A", "C", 5);
graph.addEdge("B", "C", 1);
graph.addEdge("B", "D", 3);
graph.addEdge("C", "D", 2);
graph.addEdge("C", "E", 4);
graph.addEdge("D", "E", 1);
graph.dijkstra("A");
List<Node> path = graph.getShortestPath("E");
for (Node node : path) {
System.out.print(node.name + " ");
}
}
}
```
输出结果为:A B D E
这表示从A到E的最短路径为A->B->D->E,总长度为4。
阅读全文
相关推荐
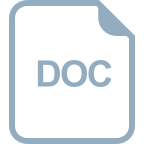
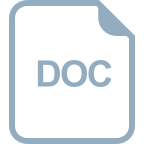
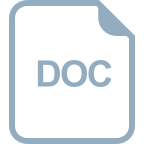
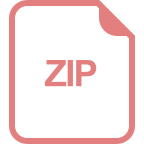
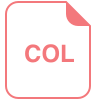

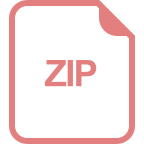
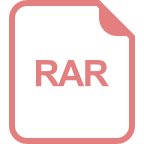

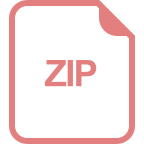
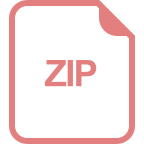
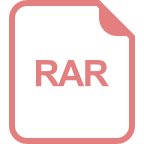
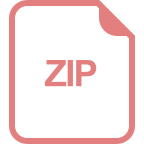
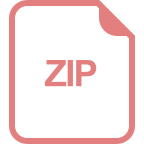
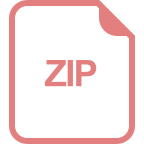
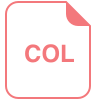
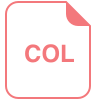

