np.random.randint(10, 25)
时间: 2023-10-13 19:19:44 浏览: 31
As an AI language model, I can generate random integers between 10 and 25 using the `numpy` library in Python. Here's the code:
```python
import numpy as np
random_int = np.random.randint(10, 25)
print(random_int)
```
This will output a random integer between 10 and 25 (inclusive).
相关问题
np.random.randint与random.randint
np.random.randint和random.randint都是用于生成随机整数的函数,但是它们有一些不同之处。
np.random.randint是numpy库中的函数,它可以生成指定范围内的随机整数,包括上限和下限。而且可以生成多个随机整数,返回一个数组。
random.randint是Python自带的随机数生成函数,它也可以生成指定范围内的随机整数,包括上限和下限。但是它只能生成一个随机整数。
下面是两个函数的使用示例:
```python
import numpy as np
import random
# 生成一个1到10之间的随机整数
a = np.random.randint(1, 11)
b = random.randint(1, 10)
print(a)
print(b)
# 生成一个1到10之间的5个随机整数
c = np.random.randint(1, 11, 5)
print(c)
```
输出结果:
```
7
9
[ 6 3 2 10 8]
```
np.random.randint np.random.random_integer
`np.random.randint` 和 `np.random.random_integer` 在 NumPy 中是没有的,可能是您写错了。但是,NumPy 中确实有两个类似的随机函数:`np.random.randint` 和 `np.random.random`。
`np.random.randint` 用于生成随机整数,语法为:
```
numpy.random.randint(low, high=None, size=None, dtype='l')
```
其中,`low` 和 `high` 分别为随机整数的最小值和最大值(左闭右开区间),`size` 为生成随机整数的数组形状,`dtype` 为数组元素的数据类型。
`np.random.random` 用于生成随机浮点数,语法为:
```
numpy.random.random(size=None)
```
其中,`size` 为随机浮点数的数组形状。
需要注意的是,`np.random.random` 生成的随机浮点数的范围是 [0,1) 的均匀分布,而 `np.random.randint` 生成的随机整数的范围是 [low, high) 的离散均匀分布。如果需要生成指定范围的随机浮点数,可以使用 `np.random.uniform` 函数。
相关推荐
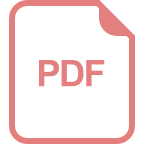
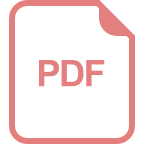
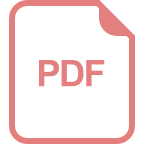











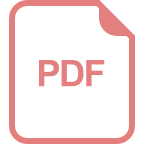
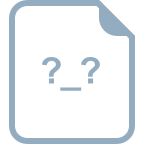
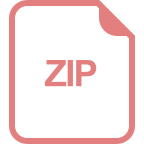