c++文件字符串的统计
时间: 2024-09-14 07:16:57 浏览: 37
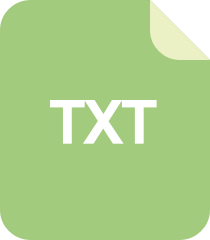
统计文件中某字符出现的次数(c++)
在C++中统计文件中的字符串数量,通常是指统计文件中单词的数量,其中单词通常由空格、标点符号等分隔。以下是一个简单的示例,展示如何使用C++进行字符串统计:
1. 首先,需要包含必要的头文件,如 `<fstream>` 用于文件操作,`<string>` 用于字符串操作。
2. 打开文件,进行读取操作。
3. 读取文件内容,通常可以逐行读取,然后对每行使用字符串流(`std::stringstream`)来分割单词。
4. 使用标准库函数如 `std::getline` 和 `std::string` 的 `find` 或 `find_first_of` 方法来查找单词之间的分隔符,并据此分割单词。
5. 对分割后的单词进行计数。
下面是一个简单的代码示例:
```cpp
#include <fstream>
#include <string>
#include <sstream>
int count_words_in_file(const std::string& file_path) {
std::ifstream file(file_path);
if (!file.is_open()) {
return -1; // 文件打开失败
}
std::string line;
int word_count = 0;
while (std::getline(file, line)) {
std::stringstream ss(line);
std::string word;
while (ss >> word) {
++word_count;
}
}
file.close();
return word_count;
}
```
在上述代码中,我们逐行读取文件内容,然后使用 `std::stringstream` 对每行进行处理,提取出单词并进行计数。最终函数返回的是文件中单词的总数。
阅读全文
相关推荐
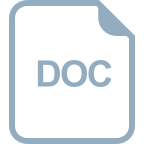
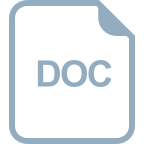
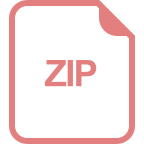
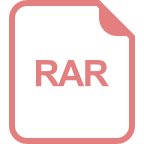
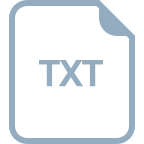
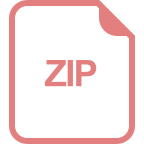
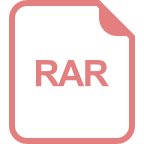
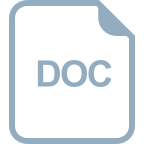









