#include<stdio.h> #define A 10 void main() { int k=0,sum=0; do{ if(k= =(k/2)*2) continue; sum+=k; } while( ++k<A); printf("%d\n",sum) ; }为什么输出结果是25
时间: 2023-11-20 12:22:18 浏览: 64
这段代码的输出结果是25,因为它是对1~9之间的奇数求和。具体来说,代码中的do-while循环从0开始迭代,每次迭代将k加1。在每次迭代中,如果k是偶数,那么continue语句会跳过当前迭代,直接进入下一次迭代。如果k是奇数,那么就将它加到sum变量中。最终,sum就是1到9之间所有奇数的和,也就是25。注意,代码中的if(k== (k/2)*2)实际上是在判断k是否为偶数,因为对于任意一个偶数k,它与自己除以2再乘以2的结果相等,而对于任意一个奇数k,这个结果就不相等了。
相关问题
//利用顺序表完成一个班级学生课程成绩的简单管理#include<stdio.h>#include<stdlib.h>#include<conio.h>#define MAXSIZE 100 //
这是一个简单的 C 语言程序片段,用于管理一个班级学生的课程成绩。它使用顺序表(如数组)作为数据结构,`MAXSIZE` 定义了列表的最大容量为 100 个元素。
```c
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#define MAXSIZE 100
// 定义顺序表结构体
typedef struct {
char name[20]; // 学生姓名
int scores[MAXSIZE]; // 课程分数数组
int count; // 当前记录数
} StudentList;
// 函数声明
void inputStudent(StudentList *list); // 输入学生信息
void displayStudent(StudentList *list); // 显示学生信息
void averageScore(StudentList *list); // 计算平均分
int main() {
StudentList studentList;
inputStudent(&studentList);
displayStudent(&studentList);
averageScore(&studentList);
return 0;
}
// 输入学生信息函数
void inputStudent(StudentList *list) {
list->count = 0;
while (list->count < MAXSIZE) {
printf("请输入学生 %d 的姓名:", list->count + 1);
fgets(list->name, sizeof(list- 1] = '\0'; // 去除换行符
printf("请输入 %d 门课程的成绩: ", MAXSIZE);
for (int i = 0; i < MAXSIZE; ++i) {
scanf("%d", &list->scores[i]);
}
++list->count;
}
}
// 显示学生信息函数
void displayStudent(StudentList *list) {
printf("\n学生信息:\n");
for (int i = 0; i < list->count; ++i) {
printf("姓名: %s\n", list->name);
printf("成绩: ");
for (int j = 0; j < MAXSIZE; ++j) {
if (list->scores[j] != 0) {
printf("%d ", list->scores[j]);
}
}
printf("\n");
}
}
// 计算平均分函数
void averageScore(StudentList *list) {
float sum = 0;
for (int i = 0; i < list->count && i < MAXSIZE; ++i) {
if (list->scores[i] != 0) {
sum += list->scores[i];
}
}
if (list->count > 0) {
printf("平均分: %.2f\n", sum / list->count);
} else {
printf("没有输入成绩。\n");
}
}
```
在这个程序中,我们创建了一个名为 `StudentList` 的结构体,其中包含了学生姓名、分数数组以及记录计数。然后定义了一些辅助函数,分别用于输入学生信息、显示学生信息以及计算平均分。主函数中先初始化学生列表,接着调用这些函数来管理学生的课程成绩。
函数接口定义: void CreateUDG(AMGraph &G); //创建图,采用邻接矩阵存储 int DFS(AMGraph G, int v);//以v为起点深度优先遍历,求出各顶点值的和作为函数返回值 裁判测试程序样例: #include <stdio.h> #include <stdlib.h> #define MVNum 100 int visited[MVNum]; typedef struct{ int vexs[MVNum]; //顶点向量,各小岛对应积分
好的,根据您提供的函数接口定义,我给出以下代码示例供参考:
```c++
#include <iostream>
#include <vector>
#include <stack>
using namespace std;
#define MVNum 100
// 邻接矩阵存储的图结构体
typedef struct {
int vexs[MVNum]; // 顶点向量,各小岛对应积分
int arcs[MVNum][MVNum]; // 邻接矩阵,记录各小岛之间的连通情况
int vexnum, arcnum; // 顶点数和边数
} AMGraph;
// 创建图,采用邻接矩阵存储
void CreateUDG(AMGraph& G) {
cout << "请输入小岛数和边数:";
cin >> G.vexnum >> G.arcnum;
cout << "请输入各小岛对应积分:";
for (int i = 0; i < G.vexnum; i++) {
cin >> G.vexs[i];
}
// 初始化邻接矩阵
for (int i = 0; i < G.vexnum; i++) {
for (int j = 0; j < G.vexnum; j++) {
G.arcs[i][j] = 0;
}
}
// 建立边
cout << "请输入各边的起点和终点:";
int v1, v2;
for (int i = 0; i < G.arcnum; i++) {
cin >> v1 >> v2;
G.arcs[v1][v2] = 1;
G.arcs[v2][v1] = 1;
}
}
// 以v为起点深度优先遍历,求出各顶点值的和作为函数返回值
int DFS(AMGraph G, int v) {
stack<int> s;
vector<int> path;
int sum = 0;
// 初始化visited数组
for (int i = 0; i < G.vexnum; i++) {
visited[i] = 0;
}
// 从起点开始遍历
s.push(v);
visited[v] = 1;
sum += G.vexs[v];
path.push_back(v);
while (!s.empty()) {
int cur = s.top();
int flag = 0;
for (int i = 0; i < G.vexnum; i++) {
if (G.arcs[cur][i] == 1 && visited[i] == 0) {
visited[i] = 1;
s.push(i);
sum += G.vexs[i];
path.push_back(i);
flag = 1;
break;
}
}
if (flag == 0) {
s.pop();
}
}
// 输出遍历路径
cout << "遍历路径为:";
for (int i = 0; i < path.size(); i++) {
cout << path[i] << " ";
}
cout << endl;
return sum;
}
int main() {
AMGraph G;
CreateUDG(G);
int sum = DFS(G, 0);
cout << "闯关成功者共获得 " << sum << " 积分" << endl;
return 0;
}
```
上述代码中,我们先定义了一个邻接矩阵存储的图结构体`AMGraph`,然后定义了`CreateUDG`和`DFS`两个函数,分别用于创建图和进行深度优先遍历计算积分。
在`DFS`函数中,我们使用了一个栈`s`来记录遍历路径,使用一个`path`列表来记录最终的遍历路径,使用一个`sum`变量来记录积分总和。我们首先将起点入栈,并将其对应的积分加入总和中,然后开始遍历。
在遍历过程中,首先取出栈顶元素,然后遍历该元素相邻的未访问过的岛屿,将其压入栈中,并将其对应的积分加入总和中。如果当前岛屿没有未访问过的相邻岛屿,说明该岛屿已经被完全遍历过了,我们将其从栈中弹出。
最后,我们将最终遍历路径输出,并返回积分总和。在`main`函数中,我们调用`CreateUDG`函数创建图,调用`DFS`函数进行深度优先遍历,并输出结果。
阅读全文
相关推荐
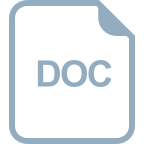
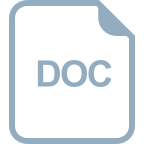
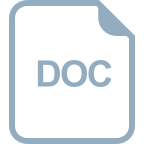














