请给以下代码注释:#include <stdio.h> #include <string.h> #include <errno.h> #include <pthread.h> #include <semaphore.h> #define SUMSIZE 100 #define BUFSIZE 8 static int bufin=0; static int bufout=0; static pthread_mutex_t buffer_lock=PTHREAD_MUTEX_INITIALIZER; static int buffer[BUFSIZE]; void put_item(int item){ pthread_mutex_lock(&buffer_lock); buffer[bufin]=item; bufin=(bufin+1)%BUFSIZE; pthread_mutex_unlock(&buffer_lock); return; } void get_item(int *itemp){ pthread_mutex_lock(&buffer_lock); *itemp=buffer[bufout]; bufout=(bufout+1)%BUFSIZE; pthread_mutex_unlock(&buffer_lock); return; } int sum=0; sem_t items; sem_t slots; static void *producer(void *); static void *consumer(void *); main(void){ pthread_t prodid; pthread_t constid; sem_init(&items,0,0); sem_init(&slots,0,BUFSIZE); pthread_create(&prodid,NULL,producer,NULL); pthread_create(&constid,NULL,consumer,NULL); pthread_join(prodid,NULL); pthread_join(constid,NULL); printf("sum=%d\n",sum); } static void *producer(void *x){ int i; for(i=1;i<=SUMSIZE;i++){ sem_wait(&slots); put_item(i); printf("Put %d\n",i); sem_post(&items); } return NULL; } static void *consumer(void *arg2){ int i,myitem; for(i=1;i<=SUMSIZE;i++){ sem_wait(&items); get_item(&myitem); printf("\tGet %d\n",myitem); sem_post(&slots); sum+=myitem; } return NULL; }
时间: 2024-03-05 22:53:06 浏览: 22
这段代码主要是一个生产者-消费者问题的解决方案,使用了线程同步和信号量的机制。下面是每个部分的具体注释:
1. 头文件包含
```
#include <stdio.h>
#include <string.h>
#include <errno.h>
#include <pthread.h>
#include <semaphore.h>
```
2. 定义常量和变量
```
#define SUMSIZE 100
#define BUFSIZE 8
static int bufin=0;
static int bufout=0;
static pthread_mutex_t buffer_lock=PTHREAD_MUTEX_INITIALIZER;
static int buffer[BUFSIZE];
```
- SUMSIZE 表示生产者和消费者一共要生产/消费的数量
- BUFSIZE 表示缓冲区的大小
- bufin 和 bufout 分别表示缓冲区的写指针和读指针
- buffer 是缓冲区数组
- buffer_lock 是互斥锁,用来保证线程安全
3. 定义函数 put_item 和 get_item
```
void put_item(int item){
pthread_mutex_lock(&buffer_lock);
buffer[bufin]=item;
bufin=(bufin+1)%BUFSIZE;
pthread_mutex_unlock(&buffer_lock);
return;
}
void get_item(int *itemp){
pthread_mutex_lock(&buffer_lock);
*itemp=buffer[bufout];
bufout=(bufout+1)%BUFSIZE;
pthread_mutex_unlock(&buffer_lock);
return;
}
```
- put_item 用来向缓冲区中写入数据
- get_item 用来从缓冲区中读取数据
4. 定义全局变量和信号量
```
int sum=0;
sem_t items;
sem_t slots;
```
- sum 表示消费者从缓冲区中读取的所有数据的和
- items 是一个信号量,用来记录缓冲区中可读的数据的个数
- slots 是一个信号量,用来记录缓冲区中可写的空间的个数
5. 定义生产者和消费者函数
```
static void *producer(void *);
static void *consumer(void *);
```
- producer 用来生产数据并写入缓冲区
- consumer 用来从缓冲区中读取数据并求和
6. 主函数
```
main(void){
pthread_t prodid;
pthread_t constid;
sem_init(&items,0,0);
sem_init(&slots,0,BUFSIZE);
pthread_create(&prodid,NULL,producer,NULL);
pthread_create(&constid,NULL,consumer,NULL);
pthread_join(prodid,NULL);
pthread_join(constid,NULL);
printf("sum=%d\n",sum);
}
```
- 创建两个线程,一个是生产者,一个是消费者
- 初始化信号量 items 和 slots
- 等待两个线程结束后,输出 sum 的值
7. 生产者函数
```
static void *producer(void *x){
int i;
for(i=1;i<=SUMSIZE;i++){
sem_wait(&slots);
put_item(i);
printf("Put %d\n",i);
sem_post(&items);
}
return NULL;
}
```
- 生产 SUMSIZE 个数据,依次写入缓冲区
- 如果缓冲区已满,就等待,直到有空间可写
- 写入数据后,通过信号量 items 通知消费者有新的数据可读
8. 消费者函数
```
static void *consumer(void *arg2){
int i,myitem;
for(i=1;i<=SUMSIZE;i++){
sem_wait(&items);
get_item(&myitem);
printf("\tGet %d\n",myitem);
sem_post(&slots);
sum+=myitem;
}
return NULL;
}
```
- 消费 SUMSIZE 个数据,依次从缓冲区中读取
- 如果缓冲区为空,就等待,直到有数据可读
- 读取数据后,通过信号量 slots 通知生产者有新的空间可写
- 将读取的数据累加到 sum 中
注:以上代码是一个简单的示例,实际的生产者-消费者问题要更为复杂,需要考虑更多的情况。
相关推荐
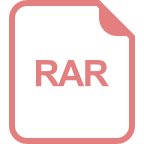
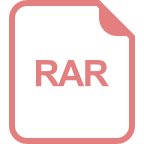
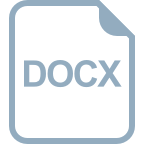















