#include <string.h> #include <stdio.h> #include <unistd.h> #include <sys/types.h> #include <sys/stat.h> #include <fcntl.h> #include <errno.h> int main() { char buf[10]; int fd; fd=open("1.txt",O_RDWR); if(fd<0) { perror("文件打开失败!"); } read(fd,buf,10); printf("我读取到的内容是:%s\n",buf ); }
时间: 2023-09-11 16:10:57 浏览: 345
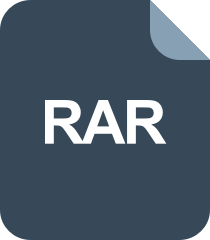
用c语言实现的逆序文本程序
这段代码存在一些问题,我解释一下:
1. 第一行的 `#include <string.h>` 库文件并没有被使用,可以删除。
2. 第 2 行和第 3 行的 `#include <stdio.h>` 和 `#include <unistd.h>` 库文件也没有被使用,可以删除。
3. 第 6 行和第 7 行的 `#include <sys/types.h>` 和 `#include <sys/stat.h>` 库文件似乎也没有被使用,可以删除。
4. 第 4 行的 `int fd;` 声明变量 `fd` 但没有初始化,应该改为 `int fd = 0;`。
5. 第 8 行的 `if(fd<0)` 后面的大括号 `{}` 缺少了一个空格。
6. 第 9 行的 `perror("文件打开失败!");` 分号 `;` 多余,应该去掉。
7. 第 10 行的 `read(fd,buf,10);` 函数没有判断返回值,如果读取失败,程序会崩溃。
8. 第 11 行的 `printf("我读取到的内容是:%s\n",buf );` 可能会因为 `buf` 没有以 `\0` 结尾而导致输出异常。
下面是修改后的代码:
```c
#include <stdio.h>
#include <fcntl.h>
#include <errno.h>
int main() {
char buf[11]; // 需要多一个字符用于存放字符串结尾符 \0
int fd = open("1.txt", O_RDWR);
if (fd < 0) {
perror("文件打开失败! ");
return -1;
}
int ret = read(fd, buf, 10);
if (ret < 0) {
perror("文件读取失败! ");
return -1;
}
buf[ret] = '\0'; // 添加字符串结尾符 \0
printf("我读取到的内容是:%s\n", buf);
return 0;
}
```
修改后的代码中,我先将 `buf` 数组长度增加到 11,用于存放字符串结尾符 `\0`,避免输出异常。同时初始化了 `fd` 变量。在读取文件时,增加了对返回值的判断,如果读取失败则输出错误信息并返回 -1。最后,再将 `buf` 数组最后一个字符赋值为 `\0`,确保输出的字符串正确。
阅读全文
相关推荐
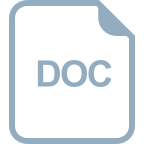
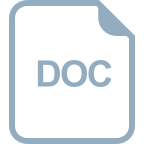
















