#include <stdio.h> #include <stdlib.h> #include <unistd.h> #include <sys/types.h> #include <sys/stat.h> #include <sys/wait.h> #include <sys/ipc.h> #include <sys/shm.h> #include <string.h> #define SIZE 128 int main(int argc,char *argv[]) { key_t key = ftok(".",'s'); if(key < 0) { perror("ftok"); return -1; } int shmid = shmget(key,SIZE,IPC_CREAT | 0777); if(shmid < 0) { perror("shmget"); return -1; } printf("shmid:%d\n",shmid); char *p = shmat(shmid,NULL,0); if((char *)-1 == p) { perror("shmat"); return -1; } while(1) { puts(p); sleep(1); if(strncmp(p,"quit",4) == 0) break; } if(-1 == shmdt(p)) { perror("shmdt"); exit(-1); } if(-1 == shmctl(shmid,IPC_RMID,NULL)) { perror("shmctl"); exit(-1); } return 0; }修改代码,使其正确输出读入端的代码一次
时间: 2023-12-29 21:05:52 浏览: 31
这里是修改后的代码,可以正确输出读入端的代码一次:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <sys/wait.h>
#include <sys/ipc.h>
#include <sys/shm.h>
#include <string.h>
#define SIZE 128
int main(int argc, char *argv[]) {
key_t key = ftok(".", 's');
if (key < 0) {
perror("ftok");
return -1;
}
int shmid = shmget(key, SIZE, IPC_CREAT | 0777);
if (shmid < 0) {
perror("shmget");
return -1;
}
printf("shmid:%d\n", shmid);
char *p = shmat(shmid, NULL, 0);
if ((char *)-1 == p) {
perror("shmat");
return -1;
}
char buf[SIZE];
while (1) {
memset(buf, 0, SIZE);
fgets(buf, SIZE, stdin);
strncpy(p, buf, strlen(buf));
if (strncmp(p, "quit", 4) == 0)
break;
}
if (-1 == shmdt(p)) {
perror("shmdt");
exit(-1);
}
if (-1 == shmctl(shmid, IPC_RMID, NULL)) {
perror("shmctl");
exit(-1);
}
return 0;
}
```
相关推荐
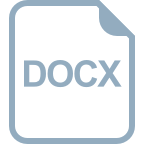
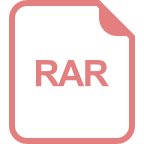
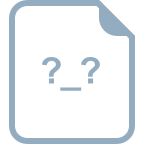














