1、顺序栈和循环队列的定义、初始化、插入和删除操作函数,并利用这些函数完成: 输入一行字符串,用户在输入错误时可以用#和@进行修正,其中#为退格符,@为退行符。要求输出修正后字符串。要求用栈作为存储结构实现。(栈和队列) 3、编写一个读入一个字符串,把它顺序读入队列,并输出队列的程序。(栈和队列)
时间: 2023-06-18 17:05:40 浏览: 136
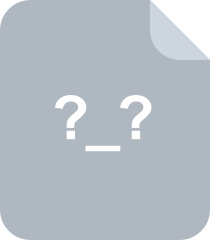
顺序循环队列的实现,包括初始化、进队,出队等
1. 顺序栈的定义、初始化、插入和删除操作函数:
```c++
#define MAXSIZE 100 // 定义栈的最大长度
typedef struct {
char data[MAXSIZE];
int top; // 栈顶指针,初始值为-1
} SqStack;
// 初始化栈
void initStack(SqStack &s) {
s.top = -1;
}
// 判断栈是否为空
bool isEmpty(SqStack s) {
return s.top == -1;
}
// 判断栈是否已满
bool isFull(SqStack s) {
return s.top == MAXSIZE - 1;
}
// 入栈操作
bool push(SqStack &s, char x) {
if (isFull(s)) {
return false;
}
s.top++;
s.data[s.top] = x;
return true;
}
// 出栈操作
bool pop(SqStack &s, char &x) {
if (isEmpty(s)) {
return false;
}
x = s.data[s.top];
s.top--;
return true;
}
```
循环队列的定义、初始化、插入和删除操作函数:
```c++
#define MAXSIZE 100 // 定义队列的最大长度
typedef struct {
char data[MAXSIZE];
int front; // 队头指针,初始值为0
int rear; // 队尾指针,初始值为0
} SqQueue;
// 初始化队列
void initQueue(SqQueue &q) {
q.front = 0;
q.rear = 0;
}
// 判断队列是否为空
bool isEmpty(SqQueue q) {
return q.front == q.rear;
}
// 判断队列是否已满
bool isFull(SqQueue q) {
return (q.rear + 1) % MAXSIZE == q.front;
}
// 入队操作
bool enQueue(SqQueue &q, char x) {
if (isFull(q)) {
return false;
}
q.data[q.rear] = x;
q.rear = (q.rear + 1) % MAXSIZE;
return true;
}
// 出队操作
bool deQueue(SqQueue &q, char &x) {
if (isEmpty(q)) {
return false;
}
x = q.data[q.front];
q.front = (q.front + 1) % MAXSIZE;
return true;
}
```
根据以上栈和队列的操作函数,实现输入一行字符串,用户在输入错误时可以用#和@进行修正,其中#为退格符,@为退行符。要求输出修正后字符串的程序:
```c++
#include <iostream>
#include <cstring>
using namespace std;
int main() {
SqStack s;
initStack(s); // 初始化栈
char c;
while (cin >> c) {
if (c == '#') { // 输入错误,退格符出栈
char x;
pop(s, x);
} else if (c == '@') { // 输入结束,输出栈中的字符
while (!isEmpty(s)) {
char x;
pop(s, x);
cout << x;
}
cout << endl;
} else { // 正常输入,入栈
push(s, c);
}
}
return 0;
}
```
编写一个读入一个字符串,把它顺序读入队列,并输出队列的程序:
```c++
#include <iostream>
#include <cstring>
using namespace std;
int main() {
SqQueue q;
initQueue(q); // 初始化队列
string str;
cin >> str;
for (int i = 0; i < str.length(); i++) { // 依次入队
enQueue(q, str[i]);
}
while (!isEmpty(q)) { // 依次出队并输出
char x;
deQueue(q, x);
cout << x;
}
cout << endl;
return 0;
}
```
阅读全文
相关推荐
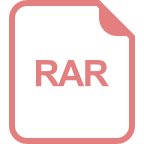





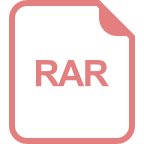
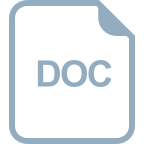
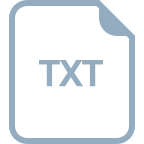
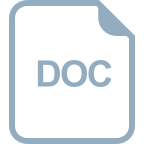
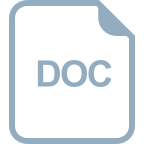
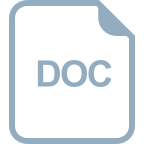
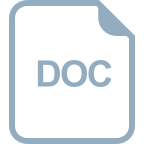
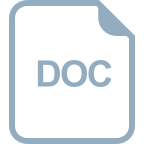



