用C语言实现,使用栈与队列逐个处理字符串中的每个字符 将line中的字符依次入栈,然后输出栈中元素个数与栈顶元素。 输入一个字符x(待删除字符)。然后将栈中元素依次出栈并输出,出栈时将不等于x的字符依次入队列,以空格分隔。 输出队列元素个数,队头与队尾,以空格分隔。 最后输出队列中所有元素。 输入格式: 输入一个个字符串 输入一个字符x(待删除字符) 输出格式: 栈中元素个数 栈顶元素 栈中符合条件的元素(以空格分隔) 队列中元素个数 队头元素 队尾元素 队列中所有元素(以空格分隔) 输入样例: ThisIsATest s 输出样例: 11 t tseTAsIsihT 8 t T teTAIihT
时间: 2024-03-08 08:47:41 浏览: 248
好的,以下是使用C语言实现的代码:
```c
#include <stdio.h>
#include <string.h>
#define MAXSIZE 100000
// 定义栈数据结构
typedef struct {
char data[MAXSIZE];
int top;
} Stack;
// 初始化栈
void init_stack(Stack *s) {
s->top = -1;
}
// 入栈
void push(Stack *s, char x) {
s->data[++s->top] = x;
}
// 出栈
char pop(Stack *s) {
if (s->top == -1) {
return '\0';
}
return s->data[s->top--];
}
// 判断栈是否为空
int is_empty(Stack *s) {
return s->top == -1;
}
// 定义队列数据结构
typedef struct {
char data[MAXSIZE];
int head;
int tail;
} Queue;
// 初始化队列
void init_queue(Queue *q) {
q->head = 0;
q->tail = -1;
}
// 入队
void enqueue(Queue *q, char x) {
q->tail++;
q->data[q->tail] = x;
}
// 出队
char dequeue(Queue *q) {
if (q->tail < q->head) {
return '\0';
}
char x = q->data[q->head];
q->head++;
return x;
}
// 判断队列是否为空
int is_queue_empty(Queue *q) {
return q->tail < q->head;
}
int main() {
char line[MAXSIZE], x;
Stack s;
Queue q;
init_stack(&s);
init_queue(&q);
// 输入字符串和待删除字符
gets(line); // gets函数可以读取含有空格的字符串
scanf("%c", &x);
// 将字符串逐个入栈
int len = strlen(line);
for (int i = 0; i < len; i++) {
push(&s, line[i]);
}
// 输出栈中元素个数和栈顶元素
printf("%d %c ", len, s.data[s.top]);
// 将栈中元素依次出栈并判断是否等于x,不等于x的字符入队
while (!is_empty(&s)) {
char c = pop(&s);
if (c != x) {
enqueue(&q, c);
}
printf("%c", c);
}
printf(" ");
// 输出队列元素个数、队头元素和队尾元素
int q_len = q.tail - q.head + 1;
printf("%d %c %c ", q_len, q.data[q.head], q.data[q.tail]);
// 输出队列中所有元素
while (!is_queue_empty(&q)) {
printf("%c", dequeue(&q));
if (!is_queue_empty(&q)) {
printf(" ");
}
}
return 0;
}
```
注意:代码中使用了gets函数来读取含有空格的字符串,这个函数比较危险,因为输入的字符串长度可能超过数组所能存储的长度,建议使用fgets函数来代替,这样可以指定最大读取字符数。
阅读全文
相关推荐
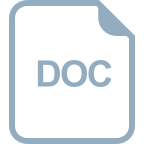
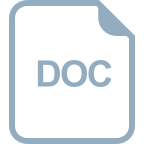
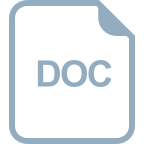

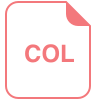
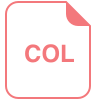
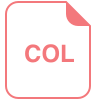
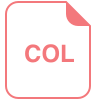
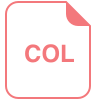
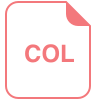
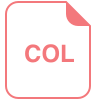
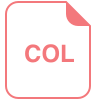
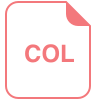
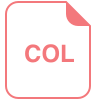
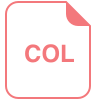
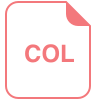
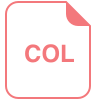
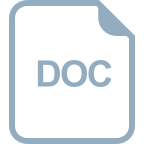
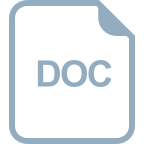