c语言实现链队列、循环队列的创建、入队、出队、获取队头元素、判断 队列是否为空、访问等运算。
时间: 2024-10-19 07:06:07 浏览: 26
在C语言中,链队列和循环队列是常用的线性数据结构,它们可以分别通过结构体和指针来实现。
1. **链队列**:
- 创建:定义一个包含数据域和两个指针(一个指向当前元素,另一个指向下一个空位置)的节点结构体。然后创建一个队列的头指针,初始设置为NULL表示队列为空。
```c
typedef struct Node {
int data;
struct Node* next;
} QueueNode;
Queue queue = { NULL, NULL };
```
- 入队(enqueue):在队尾添加新元素。如果队列为空,新建节点并设置头指针;否则,直接将新节点链接到当前尾部。
```c
void enqueue(Queue *queue, int value) {
QueueNode* newNode = (QueueNode*)malloc(sizeof(QueueNode));
newNode->data = value;
newNode->next = queue->tail;
if (queue->head == NULL)
queue->head = queue->tail = newNode;
else
queue->tail->next = newNode;
queue->tail = newNode;
}
```
- 出队(dequeue):移除队首元素,并更新头指针。若队列为空则返回错误。
```c
int dequeue(Queue *queue) {
if (queue->head == NULL)
return -1; // 队列为空
int value = queue->head->data;
QueueNode* temp = queue->head;
queue->head = queue->head->next;
free(temp);
if (queue->head == NULL)
queue->tail = NULL;
return value;
}
```
- 获取队头元素(front):只读操作,队列非空的情况下返回队首元素值。
```c
int front(const Queue *queue) {
return queue->head ? queue->head->data : -1;
}
```
- 判断队列是否为空(is_empty):检查头指针是否为NULL。
```c
int is_empty(const Queue *queue) {
return queue->head == NULL;
}
```
2. **循环队列**:
- 创建类似链队列,但在底层实现上是连续内存空间。需要额外的索引变量维护队尾的位置。
```c
#define QUEUE_SIZE 10
int queue[QUEUE_SIZE];
int front = 0, rear = -1;
```
- 入队:计算新的rear,如果超过数组大小减去1,就从front开始重置。
```c
void enqueue(int value) {
if ((rear + 1) % QUEUE_SIZE == front) // 当队满
rear = front; // 重置 rear
queue[++rear] = value;
}
```
- 出队:更新front,如果front等于rear,则表示队列已空。
```c
int dequeue() {
if (front == rear) // 队列空
return -1;
int value = queue[front++];
if (front > rear)
front = 0; // 向前移动front
return value;
}
```
- 其他操作类似链队列,只需相应地修改队首和队尾的处理即可。
阅读全文
相关推荐
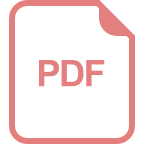
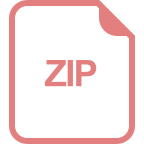


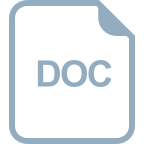
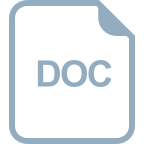
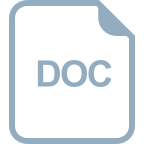
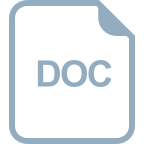









