使用C语言写一段代码: 给定n个实数,求这n个数在实轴上相邻两个数之间的最大差值。要先从小到大进行排序,再求最大间隙 如有5个实数: 2.3, 3.1, 7.5, 1.5, 6.3 则最大间隙为: 3.2
时间: 2024-09-07 21:01:19 浏览: 69
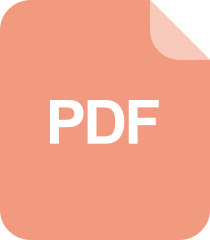
固定轴法的基本原理-走出软件作坊_阿朱(pdf高清文字版)
以下是使用C语言编写的一段代码,用于解决这个问题:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义函数来计算最大间隙
double findMaxGap(int arr[], int n) {
// 对数组进行排序
qsort(arr, n, sizeof(double), compare);
// 初始化最大间隙为第一个元素和第二个元素的差
double maxGap = arr[1] - arr[0];
// 遍历数组,找到每个元素与其下一个元素的差,并更新最大间隙
for (int i = 1; i < n - 1; i++) {
if (arr[i+1] - arr[i] > maxGap)
maxGap = arr[i+1] - arr[i];
}
return maxGap;
}
// 比较函数,用于qsort对数组进行升序排列
int compare(const void *a, const void *b) {
return (*(double*)a - *(double*)b);
}
int main() {
double numbers[] = {2.3, 3.1, 7.5, 1.5, 6.3};
int n = sizeof(numbers) / sizeof(numbers[0]);
printf("The maximum gap is: %lf\n", findMaxGap(numbers, n));
return 0;
}
```
这段代码首先包含了必要的头文件,然后定义了一个`findMaxGap`函数,它接收一个双精度浮点数数组和数组长度。通过`qsort`函数对数组进行排序,然后遍历数组找出最大的间隔。在`main`函数中,我们创建了一个包含五个给定实数的数组,并调用`findMaxGap`函数计算结果。
阅读全文
相关推荐
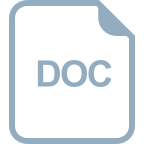
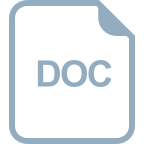

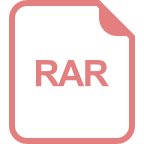


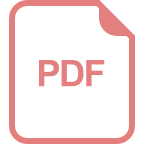
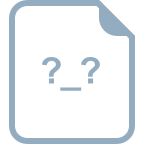
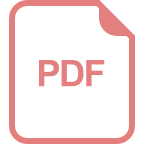
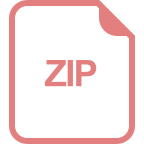
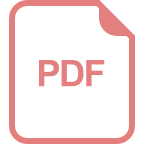
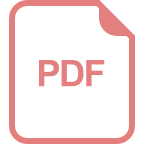
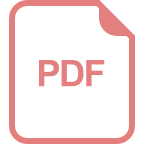
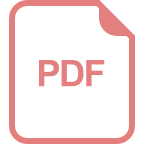
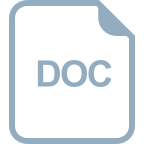
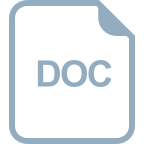
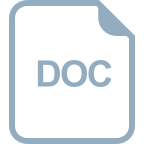
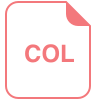