解题思路Java代码编写一个实验程序.假设二叉树采用二叉链存储结构,所有结点值为单个字符且不相同。采用先序遍历、非递归后序遍历和层次遍历方式输出二叉树中从根结点到每个叶子 结点的路径,并且利用图7.17进行测试。
时间: 2023-06-05 19:06:32 浏览: 218
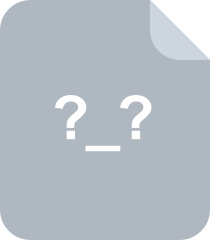
JAVA 二叉树实验
好的,关于你的问题,我可以回答。你需要编写一个实验程序,输出二叉树中从根结点到每个叶子结点的路径。这个程序采用二叉链存储结构,所有结点值为单个字符且不相同,并且使用先序遍历、非递归后序遍历和层次遍历方式进行遍历输出。
以下是 Java 代码实现:
```java
// 定义二叉树结点
class TreeNode {
char val;
TreeNode left, right;
public TreeNode(char c) {
val = c;
left = right = null;
}
}
// 主类
public class BinaryTree {
TreeNode root;
// 构造函数,创建二叉树
public BinaryTree() {
// 创建图7.17中的二叉树
root = new TreeNode('B');
root.left = new TreeNode('A');
root.right = new TreeNode('D');
root.right.left = new TreeNode('C');
root.right.right = new TreeNode('E');
}
// 先序遍历
public void preOrder(TreeNode node, String path) {
if (node == null) {
return;
}
// 当前节点为叶子结点,输出路径
if (node.left == null && node.right == null) {
System.out.println("Path: " + path + node.val);
} else {
// 非叶子结点,递归左右子节点
preOrder(node.left, path + node.val + "->");
preOrder(node.right, path + node.val + "->");
}
}
// 非递归后序遍历
public void postOrder(TreeNode node) {
if (node == null) {
return;
}
Stack<TreeNode> s1 = new Stack<>();
Stack<Character> s2 = new Stack<>();
s1.push(node);
while (!s1.isEmpty()) {
TreeNode temp = s1.pop();
s2.push(temp.val);
if (temp.left != null) {
s1.push(temp.left);
}
if (temp.right != null) {
s1.push(temp.right);
}
}
while (!s2.isEmpty()) {
System.out.println("Path: " + getPath(node, s2.pop()));
}
}
// 获取节点到根节点的路径
public String getPath(TreeNode node, char c) {
if (node == null) {
return "";
}
if (node.val == c) {
return "" + node.val;
}
String leftPath = getPath(node.left, c);
if (!leftPath.equals("")) {
return leftPath + "->" + node.val;
}
String rightPath = getPath(node.right, c);
if (!rightPath.equals("")) {
return rightPath + "->" + node.val;
}
return "";
}
// 层次遍历
public void levelOrder(TreeNode node) {
if (node == null) {
return;
}
Queue<TreeNode> queue = new LinkedList<>();
queue.offer(node);
while (!queue.isEmpty()) {
int size = queue.size();
for (int i = 0; i < size; i++) {
TreeNode temp = queue.poll();
// 叶子结点,输出路径
if (temp.left == null && temp.right == null) {
System.out.println("Path: " + getPath(node, temp.val));
}
if (temp.left != null) {
queue.offer(temp.left);
}
if (temp.right != null) {
queue.offer(temp.right);
}
}
}
}
// 测试
public static void main(String[] args) {
BinaryTree tree = new BinaryTree();
tree.preOrder(tree.root, "");
tree.postOrder(tree.root);
tree.levelOrder(tree.root);
}
}
```
这个程序采用的是三种不同的遍历方式,先序遍历、非递归后序遍历和层次遍历,以输出二叉树中从根结点到每个叶子结点的路径。
阅读全文
相关推荐
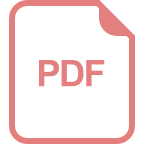














