NV21和RGB互相转换的工具
时间: 2024-04-30 11:21:47 浏览: 11
可以使用Java或C++等编程语言来实现NV21和RGB之间的转换。以下是一个Java实现的示例代码:
```java
public class ImageUtil {
/**
* 将NV21格式的字节数组转换为RGB格式的字节数组
* @param nv21Byte NV21格式的字节数组
* @param width 图像宽度
* @param height 图像高度
* @return RGB格式的字节数组
*/
public static byte[] nv21ToRgb(byte[] nv21Byte, int width, int height) {
byte[] rgbByte = new byte[width * height * 3];
int nvPixelCount = width * height;
int uvIndex = nvPixelCount;
int rIndex, gIndex, bIndex, yIndex, uIndex, vIndex;
int y, u, v, r, g, b;
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
yIndex = i * width + j;
uIndex = uvIndex + (i / 2) * width + (j / 2) * 2;
vIndex = uvIndex + (i / 2) * width + (j / 2) * 2 + 1;
y = nv21Byte[yIndex] & 0xff;
u = nv21Byte[uIndex] & 0xff;
v = nv21Byte[vIndex] & 0xff;
r = (int)(y + 1.370705f * (v - 128));
g = (int)(y - 0.698001f * (v - 128) - 0.337633f * (u - 128));
b = (int)(y + 1.732446f * (u - 128));
r = Math.max(0, Math.min(255, r));
g = Math.max(0, Math.min(255, g));
b = Math.max(0, Math.min(255, b));
rIndex = (i * width + j) * 3;
gIndex = (i * width + j) * 3 + 1;
bIndex = (i * width + j) * 3 + 2;
rgbByte[rIndex] = (byte)r;
rgbByte[gIndex] = (byte)g;
rgbByte[bIndex] = (byte)b;
}
}
return rgbByte;
}
/**
* 将RGB格式的字节数组转换为NV21格式的字节数组
* @param rgbByte RGB格式的字节数组
* @param width 图像宽度
* @param height 图像高度
* @return NV21格式的字节数组
*/
public static byte[] rgbToNv21(byte[] rgbByte, int width, int height) {
byte[] nv21Byte = new byte[width * height * 3 / 2];
int nvPixelCount = width * height;
int uvIndex = nvPixelCount;
int rIndex, gIndex, bIndex, yIndex, uIndex, vIndex;
int y, u, v, r, g, b;
for (int i = 0; i < height; i++) {
for (int j = 0; j < width; j++) {
rIndex = (i * width + j) * 3;
gIndex = (i * width + j) * 3 + 1;
bIndex = (i * width + j) * 3 + 2;
r = rgbByte[rIndex] & 0xff;
g = rgbByte[gIndex] & 0xff;
b = rgbByte[bIndex] & 0xff;
y = (int)(0.299 * r + 0.587 * g + 0.114 * b);
u = (int)(-0.14713 * r - 0.28886 * g + 0.436 * b + 128);
v = (int)(0.615 * r - 0.51499 * g - 0.10001 * b + 128);
y = Math.max(0, Math.min(255, y));
u = Math.max(0, Math.min(255, u));
v = Math.max(0, Math.min(255, v));
yIndex = i * width + j;
uIndex = uvIndex + (i / 2) * width + (j / 2) * 2;
vIndex = uvIndex + (i / 2) * width + (j / 2) * 2 + 1;
nv21Byte[yIndex] = (byte)y;
nv21Byte[uIndex] = (byte)u;
nv21Byte[vIndex] = (byte)v;
}
}
return nv21Byte;
}
}
```
其中,nv21ToRgb()方法将NV21格式的字节数组转换为RGB格式的字节数组,rgbToNv21()方法将RGB格式的字节数组转换为NV21格式的字节数组。具体使用方法可以根据实际情况进行调整。
相关推荐
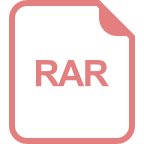














