pytest 和conftest 结合使用
时间: 2023-07-08 15:13:11 浏览: 104
在 pytest 中,conftest.py 文件是一个特殊的文件,用于定义测试用例之间共享的 fixture 函数。fixture 函数可以在测试用例中通过名称来调用,这样可以避免重复编写代码。下面是 pytest 和 conftest 结合使用的示例:
1. 创建一个 conftest.py 文件,定义一个 fixture 函数:
```python
import pytest
@pytest.fixture(scope='session')
def login():
print("登录系统")
yield
print("退出系统")
```
2. 创建一个测试用例文件 test_demo.py,使用 conftest.py 中定义的 fixture 函数:
```python
def test_case1(login):
print("执行测试用例1")
def test_case2(login):
print("执行测试用例2")
```
在执行测试用例时,pytest 会自动查找 conftest.py 文件中定义的 fixture 函数,并将其传递给测试用例。在上面的示例中,每个测试用例都会先执行 login 函数,然后再执行测试用例本身。执行顺序如下:
```
登录系统
执行测试用例1
退出系统
登录系统
执行测试用例2
退出系统
```
这样可以确保每个测试用例都在同一个环境下执行,避免了测试用例之间的干扰。同时,也避免了重复编写登录和退出系统等代码的问题。
相关问题
实现一个代码框架,要求使用 conftest和pytest fixture 结合实现变量的使用,不同的变量作用域
好的,以下是一个示例代码框架,使用了 `conftest` 和 `pytest fixture`,并实现了不同作用域的变量使用:
conftest.py
```python
import pytest
# session 级别的 fixture,作用域为整个测试会话
@pytest.fixture(scope='session')
def session_fixture():
print('session fixture setup')
yield
print('session fixture teardown')
# module 级别的 fixture,作用域为当前测试模块
@pytest.fixture(scope='module')
def module_fixture():
print('module fixture setup')
yield
print('module fixture teardown')
# function 级别的 fixture,作用域为每个测试函数
@pytest.fixture(scope='function')
def function_fixture():
print('function fixture setup')
yield
print('function fixture teardown')
```
test_example.py
```python
def test_one(session_fixture, module_fixture, function_fixture):
print('test one')
def test_two(session_fixture, module_fixture, function_fixture):
print('test two')
```
在上面的代码中,我们定义了三个不同作用域的 fixture,分别是 `session_fixture`、`module_fixture` 和 `function_fixture`。在 `test_example.py` 中,我们使用了这三个 fixture,每个测试函数都会执行这三个 fixture,且每个 fixture 的作用域不同。
如果我们执行 `pytest test_example.py` 命令,输出如下:
```
session fixture setup
module fixture setup
function fixture setup
test one
function fixture teardown
function fixture setup
test two
function fixture teardown
module fixture teardown
session fixture teardown
```
可以看到,`session_fixture` 在整个测试会话中只会执行一次,在测试开始前和结束后分别执行了 `session_fixture setup` 和 `session_fixture teardown`。`module_fixture` 在每个测试模块中执行一次,在测试模块开始前和结束后分别执行了 `module_fixture setup` 和 `module_fixture teardown`。`function_fixture` 在每个测试函数中执行一次,在每个测试函数开始前和结束后分别执行了 `function_fixture setup` 和 `function_fixture teardown`。
pytest自动化框架,就conftest配置文件、common中间层公共方法、测试用例、数据驱动、allure、pytest、fixture、logs,写一份操作pytest测试框架使用介绍指南?
好的,下面是使用pytest测试框架的一些指南:
1. 安装pytest
使用pip安装pytest模块:
```
pip install pytest
```
2. 创建测试用例
在项目目录中创建一个名为`test_*.py`(*代表任意字符)的测试用例文件,例如:
```
# test_math.py
def test_add():
assert 1 + 1 == 2
def test_subtract():
assert 2 - 1 == 1
```
3. 运行测试用例
在命令行中进入项目目录,执行以下命令:
```
pytest
```
pytest会自动识别当前目录下的测试用例文件,并执行其中的测试用例。
4. 使用fixture
fixture是pytest中非常重要的一个概念,它可以提供一些测试用例所需要的前置操作或者后置操作。例如:
```
# conftest.py
import pytest
@pytest.fixture
def setup():
print("执行测试用例前的准备工作")
yield
print("执行测试用例后的清理工作")
```
```
# test_math.py
def test_add(setup):
assert 1 + 1 == 2
def test_subtract(setup):
assert 2 - 1 == 1
```
在测试用例中使用fixture时,需要在测试用例的参数中指定fixture的名称,例如:`def test_add(setup):`。
5. 参数化
pytest支持参数化测试,可以通过`@pytest.mark.parametrize`装饰器来实现。例如:
```
# test_math.py
import pytest
@pytest.mark.parametrize("a,b,result", [(1, 1, 2), (2, 1, 1), (0, 0, 0)])
def test_add(a, b, result):
assert a + b == result
```
6. 使用allure生成报告
pytest可以结合allure生成漂亮的测试报告。安装allure命令行工具:
```
brew install allure
```
在pytest中使用allure时,需要安装pytest-allure插件:
```
pip install pytest-allure
```
使用allure生成测试报告:
```
pytest --alluredir=./report
allure serve ./report
```
7. 编写中间层公共方法
在项目中,如果有多个测试用例需要用到同一个方法,可以将这些方法放在一个common.py中。例如:
```
# common.py
def login(username, password):
print("登录操作")
```
在测试用例中使用common.py中的方法:
```
# test_math.py
from common import login
def test_add():
login("admin", "123456")
assert 1 + 1 == 2
```
8. 使用日志记录测试过程
在测试过程中,可以使用logging模块记录日志。例如:
```
# test_math.py
import logging
def test_add():
logging.info("执行加法测试用例")
assert 1 + 1 == 2
```
可以通过设置logging的level来控制日志的输出级别。
这就是使用pytest测试框架的一些指南。希望能够对你有所帮助!
阅读全文
相关推荐
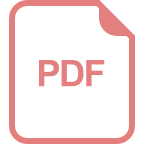
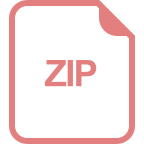
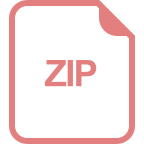
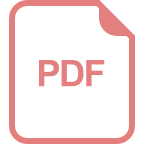
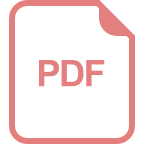
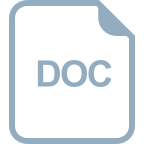
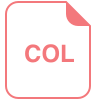
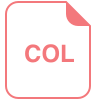
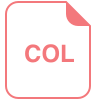
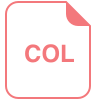
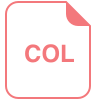
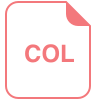
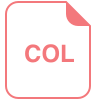
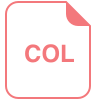
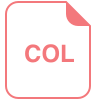
