# 导入数据集 def import_csv_data(): global file_path, df, txt_data file_path = filedialog.askopenfilename() df = pd.read_csv(file_path) df = df.fillna(0) top_5 = df.head() txt_data.delete('1.0', tk.END) txt_data.insert(tk.END, top_5) # 处理缺失值 def handle_missing_values(): global file_path # 读取CSV文件 data = pd.read_csv(file_path) # 处理缺失值 data.fillna(0, inplace=True) # 显示前10行数据 text_output.insert(tk.END, "处理缺失值成功,前10行数据如下:\n") text_output.insert(tk.END, str(data.head(10))) # 标准化数值型数据 def normalize_numeric_data(): global file_path # 读取CSV文件 data = pd.read_csv(file_path) # 提取数值型数据 numeric_data = data.select_dtypes(include=['float64', 'int64']) # 标准化数据 scaler = StandardScaler() normalized_data = scaler.fit_transform(numeric_data) # 将处理后的数据写回原数据框 data.loc[:, numeric_data.columns] = normalized_data # 显示前10行数据 text_output.insert(tk.END, "标准化数值型数据成功,前10行数据如下:\n") text_output.insert(tk.END, str(data.head(10))) # 划分训练集和测试集 def split_train_test(): global file_path, train_ratio # 读取CSV文件 data = pd.read_csv(file_path) # 划分数据集 train, test = train_test_split(data, train_size=train_ratio) # 显示训练集和测试集大小 text_output.insert(tk.END, "训练集大小:{}\n".format(len(train))) text_output.insert(tk.END, "测试集大小:{}\n".format(len(test)))续写代码实现“模型下拉菜单,可选择相应模型,选择后,对相应模型进行训练,测试”的功能
时间: 2024-02-22 08:57:51 浏览: 120
在原有代码的基础上,可以添加一个下拉菜单组件,用于选择机器学习模型,以及相应的训练和测试函数。具体代码如下:
```
# 导入机器学习模型库
from sklearn.linear_model import LinearRegression
from sklearn.tree import DecisionTreeRegressor
from sklearn.ensemble import RandomForestRegressor
# 创建下拉菜单组件,用于选择机器学习模型
model_var = tk.StringVar(root)
model_options = ["线性回归", "决策树", "随机森林"]
model_var.set(model_options[0])
model_menu = tk.OptionMenu(root, model_var, *model_options)
model_menu.pack()
# 创建训练和测试函数
def train_linear_regression():
global df, train_ratio
# 划分训练集和测试集
x_train, x_test, y_train, y_test = train_test_split(df.iloc[:, :-1], df.iloc[:, -1], train_size=train_ratio)
# 训练线性回归模型
model = LinearRegression()
model.fit(x_train, y_train)
# 测试模型
score = model.score(x_test, y_test)
# 显示模型评分
text_output.insert(tk.END, "线性回归模型测试评分:{:.2f}\n".format(score))
def train_decision_tree():
global df, train_ratio
# 划分训练集和测试集
x_train, x_test, y_train, y_test = train_test_split(df.iloc[:, :-1], df.iloc[:, -1], train_size=train_ratio)
# 训练决策树模型
model = DecisionTreeRegressor()
model.fit(x_train, y_train)
# 测试模型
score = model.score(x_test, y_test)
# 显示模型评分
text_output.insert(tk.END, "决策树模型测试评分:{:.2f}\n".format(score))
def train_random_forest():
global df, train_ratio
# 划分训练集和测试集
x_train, x_test, y_train, y_test = train_test_split(df.iloc[:, :-1], df.iloc[:, -1], train_size=train_ratio)
# 训练随机森林模型
model = RandomForestRegressor()
model.fit(x_train, y_train)
# 测试模型
score = model.score(x_test, y_test)
# 显示模型评分
text_output.insert(tk.END, "随机森林模型测试评分:{:.2f}\n".format(score))
# 创建训练和测试按钮
train_button = tk.Button(root, text="训练和测试", command=train_selected_model)
train_button.pack()
# 创建训练和测试函数
def train_selected_model():
global model_var
selected_model = model_var.get()
if selected_model == "线性回归":
train_linear_regression()
elif selected_model == "决策树":
train_decision_tree()
elif selected_model == "随机森林":
train_random_forest()
```
在这段代码中,我们首先创建了一个下拉菜单组件,并提供了三个机器学习模型供选择。然后,我们创建了三个训练和测试函数,分别对应线性回归、决策树和随机森林模型。最后,我们创建了一个按钮,用于触发训练和测试函数。在按钮的回调函数中,我们获取了下拉菜单中选择的模型,并根据选择的模型调用相应的训练和测试函数。
阅读全文
相关推荐
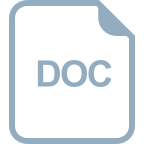
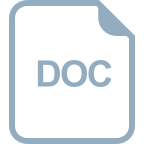
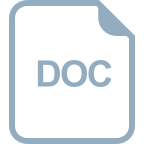














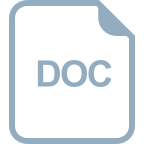
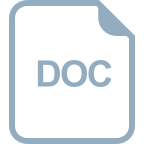