import torch import os import torch.nn as nn import torch.optim as optim import numpy as np import random class Net(nn.Module): def init(self): super(Net, self).init() self.conv1 = nn.Conv2d(1, 16, kernel_size=3,stride=1) self.pool = nn.MaxPool2d(kernel_size=2,stride=2) self.conv2 = nn.Conv2d(16, 32, kernel_size=3,stride=1) self.fc1 = nn.Linear(32 * 9 * 9, 120) self.fc2 = nn.Linear(120, 84) self.fc3 = nn.Linear(84, 2) def forward(self, x): x = self.pool(nn.functional.relu(self.conv1(x))) x = self.pool(nn.functional.relu(self.conv2(x))) x = x.view(-1, 32 * 9 * 9) x = nn.functional.relu(self.fc1(x)) x = nn.functional.relu(self.fc2(x)) x = self.fc3(x) return x net = Net() criterion = nn.CrossEntropyLoss() optimizer = optim.SGD(net.parameters(), lr=0.001, momentum=0.9) folder_path = 'random_matrices2' # 创建空的tensor x = torch.empty((40, 1, 42, 42)) # 遍历文件夹内的文件,将每个矩阵转化为tensor并存储 for j in range(40): for j in range(40): file_name = 'matrix_{}.npy'.format(j) file_path = os.path.join(folder_path, file_name) matrix = np.load(file_path) x[j] = torch.from_numpy(matrix).unsqueeze(0) #y = torch.cat((torch.zeros(20), torch.ones(20))) y = torch.cat((torch.zeros(20, dtype=torch.long), torch.ones(20, dtype=torch.long))) for epoch in range(10): running_loss = 0.0 for i in range(40): inputs = x[i] labels = y[i] optimizer.zero_grad() outputs = net(inputs) #loss = criterion(outputs, labels) loss = criterion(outputs.unsqueeze(0), labels.unsqueeze(0)) loss.backward() optimizer.step() running_loss += loss.item() print('[%d] loss: %.3f' % (epoch + 1, running_loss / 40)) print('Finished Training') 上述代码报错:RuntimeError: Expected target size [1, 2], got [1],怎么修改?
时间: 2023-11-29 16:07:58 浏览: 116
这个错误是因为标签 `labels` 的大小为 `[1]`,而交叉熵损失函数 `nn.CrossEntropyLoss()` 需要一个大小为 `[1, 2]` 的标签张量。可以通过调用 `unsqueeze()` 函数来将标签张量的大小从 `[1]` 转换为 `[1, 1]`,然后再使用 `torch.nn.functional.one_hot()` 将标签张量转换为独热编码格式,大小为 `[1, 2]`。修改后的代码如下:
```
import torch
import os
import torch.nn as nn
import torch.optim as optim
import numpy as np
import random
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.conv1 = nn.Conv2d(1, 16, kernel_size=3,stride=1)
self.pool = nn.MaxPool2d(kernel_size=2,stride=2)
self.conv2 = nn.Conv2d(16, 32, kernel_size=3,stride=1)
self.fc1 = nn.Linear(32 * 9 * 9, 120)
self.fc2 = nn.Linear(120, 84)
self.fc3 = nn.Linear(84, 2)
def forward(self, x):
x = self.pool(nn.functional.relu(self.conv1(x)))
x = self.pool(nn.functional.relu(self.conv2(x)))
x = x.view(-1, 32 * 9 * 9)
x = nn.functional.relu(self.fc1(x))
x = nn.functional.relu(self.fc2(x))
x = self.fc3(x)
return x
net = Net()
criterion = nn.CrossEntropyLoss()
optimizer = optim.SGD(net.parameters(), lr=0.001, momentum=0.9)
folder_path = 'random_matrices2'
# 创建空的tensor
x = torch.empty((40, 1, 42, 42))
# 遍历文件夹内的文件,将每个矩阵转化为tensor并存储
for j in range(40):
file_name = 'matrix_{}.npy'.format(j)
file_path = os.path.join(folder_path, file_name)
matrix = np.load(file_path)
x[j] = torch.from_numpy(matrix).unsqueeze(0)
y = torch.cat((torch.zeros(20, dtype=torch.long), torch.ones(20, dtype=torch.long)))
for epoch in range(10):
running_loss = 0.0
for i in range(40):
inputs = x[i]
labels = y[i].unsqueeze(0)
labels = nn.functional.one_hot(labels, num_classes=2)
optimizer.zero_grad()
outputs = net(inputs)
loss = criterion(outputs.unsqueeze(0), labels.float())
loss.backward()
optimizer.step()
running_loss += loss.item()
print('[%d] loss: %.3f' % (epoch + 1, running_loss / 40))
print('Finished Training')
```
阅读全文
相关推荐
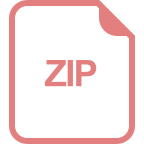
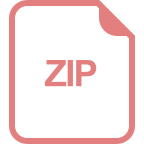
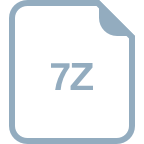














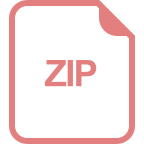
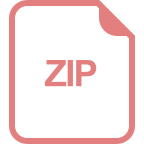