vivado HDL实现VGA显示白底黑字“FPGA”
时间: 2023-09-22 14:07:55 浏览: 48
要在VGA上显示“FPGA”字符,需要使用VHDL或Verilog编写代码来生成HDL模块,并使用Vivado工具将其综合到FPGA芯片中。以下是示例VHDL代码:
```
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
use IEEE.STD_LOGIC_UNSIGNED.ALL;
use IEEE.NUMERIC_STD.ALL;
entity VGA_display is
Port ( clk : in STD_LOGIC;
reset : in STD_LOGIC;
hsync : out STD_LOGIC;
vsync : out STD_LOGIC;
r : out STD_LOGIC_VECTOR (3 downto 0);
g : out STD_LOGIC_VECTOR (3 downto 0);
b : out STD_LOGIC_VECTOR (3 downto 0));
end VGA_display;
architecture Behavioral of VGA_display is
-- VGA timing parameters
constant H_DISPLAY : integer := 640;
constant H_FRONT_PORCH : integer := 16;
constant H_SYNC : integer := 96;
constant H_BACK_PORCH : integer := 48;
constant V_DISPLAY : integer := 480;
constant V_FRONT_PORCH : integer := 10;
constant V_SYNC : integer := 2;
constant V_BACK_PORCH : integer := 33;
-- Counters
signal hcount : integer range 0 to H_DISPLAY+H_FRONT_PORCH+H_SYNC+H_BACK_PORCH-1 := 0;
signal vcount : integer range 0 to V_DISPLAY+V_FRONT_PORCH+V_SYNC+V_BACK_PORCH-1 := 0;
-- VGA colors
constant BLACK : std_logic_vector(3 downto 0) := "0000";
constant WHITE : std_logic_vector(3 downto 0) := "1111";
-- Display character "FPGA"
constant F : std_logic_vector(6 downto 0) := "0111111";
constant P : std_logic_vector(6 downto 0) := "1111001";
constant G : std_logic_vector(6 downto 0) := "1011011";
constant A : std_logic_vector(6 downto 0) := "1110111";
-- Display buffer
signal buffer : std_logic_vector(31 downto 0) := (others => '0');
begin
process (clk, reset)
begin
if (reset = '1') then
hcount <= 0;
vcount <= 0;
buffer <= (others => '0');
elsif (rising_edge(clk)) then
-- Horizontal counter
if (hcount = H_DISPLAY+H_FRONT_PORCH+H_SYNC+H_BACK_PORCH-1) then
hcount <= 0;
-- Vertical counter
if (vcount = V_DISPLAY+V_FRONT_PORCH+V_SYNC+V_BACK_PORCH-1) then
vcount <= 0;
else
vcount <= vcount + 1;
end if;
else
hcount <= hcount + 1;
end if;
end if;
end process;
-- Generate VGA signals
hsync <= '1' when ((hcount >= H_DISPLAY+H_FRONT_PORCH) and (hcount < H_DISPLAY+H_FRONT_PORCH+H_SYNC)) else '0';
vsync <= '1' when ((vcount >= V_DISPLAY+V_FRONT_PORCH) and (vcount < V_DISPLAY+V_FRONT_PORCH+V_SYNC)) else '0';
-- Generate display buffer
process (clk)
begin
if (rising_edge(clk)) then
case hcount is
when 0 to 7 =>
buffer <= F & BLACK & BLACK & BLACK;
when 8 to 15 =>
buffer <= BLACK & F & BLACK & BLACK;
when 16 to 23 =>
buffer <= BLACK & BLACK & F & BLACK;
when 24 to 31 =>
buffer <= BLACK & BLACK & BLACK & F;
when H_DISPLAY+H_FRONT_PORCH+H_SYNC to H_DISPLAY+H_FRONT_PORCH+H_SYNC+7 =>
buffer <= P & BLACK & BLACK & BLACK;
when H_DISPLAY+H_FRONT_PORCH+H_SYNC+8 to H_DISPLAY+H_FRONT_PORCH+H_SYNC+15 =>
buffer <= BLACK & P & BLACK & BLACK;
when H_DISPLAY+H_FRONT_PORCH+H_SYNC+16 to H_DISPLAY+H_FRONT_PORCH+H_SYNC+23 =>
buffer <= BLACK & BLACK & P & BLACK;
when H_DISPLAY+H_FRONT_PORCH+H_SYNC+24 to H_DISPLAY+H_FRONT_PORCH+H_SYNC+31 =>
buffer <= BLACK & BLACK & BLACK & P;
when H_DISPLAY+H_FRONT_PORCH+H_SYNC+32 to H_DISPLAY+H_FRONT_PORCH+H_SYNC+39 =>
buffer <= G & BLACK & BLACK & BLACK;
when H_DISPLAY+H_FRONT_PORCH+H_SYNC+40 to H_DISPLAY+H_FRONT_PORCH+H_SYNC+47 =>
buffer <= BLACK & G & BLACK & BLACK;
when H_DISPLAY+H_FRONT_PORCH+H_SYNC+48 to H_DISPLAY+H_FRONT_PORCH+H_SYNC+55 =>
buffer <= BLACK & BLACK & G & BLACK;
when H_DISPLAY+H_FRONT_PORCH+H_SYNC+56 to H_DISPLAY+H_FRONT_PORCH+H_SYNC+63 =>
buffer <= BLACK & BLACK & BLACK & G;
when H_DISPLAY+H_FRONT_PORCH+H_SYNC+64 to H_DISPLAY+H_FRONT_PORCH+H_SYNC+71 =>
buffer <= A & BLACK & BLACK & BLACK;
when H_DISPLAY+H_FRONT_PORCH+H_SYNC+72 to H_DISPLAY+H_FRONT_PORCH+H_SYNC+79 =>
buffer <= BLACK & A & BLACK & BLACK;
when H_DISPLAY+H_FRONT_PORCH+H_SYNC+80 to H_DISPLAY+H_FRONT_PORCH+H_SYNC+87 =>
buffer <= BLACK & BLACK & A & BLACK;
when H_DISPLAY+H_FRONT_PORCH+H_SYNC+88 to H_DISPLAY+H_FRONT_PORCH+H_SYNC+95 =>
buffer <= BLACK & BLACK & BLACK & A;
when others =>
buffer <= (others => '0');
end case;
end if;
end process;
-- Output VGA colors
r <= buffer(31 downto 28);
g <= buffer(27 downto 24);
b <= buffer(23 downto 20);
end Behavioral;
```
在这个示例中,我们使用了一个计数器来控制VGA信号的生成,并使用一个缓冲区来生成要在屏幕上显示的像素数据。我们将HDL模块的输出连接到FPGA芯片的VGA引脚上。在每个时钟周期内,我们根据计数器的值来确定当前的像素所应该显示的颜色。在这个示例中,我们使用了四种颜色:黑色、白色、红色和绿色,来显示“FPGA”字符。
相关推荐
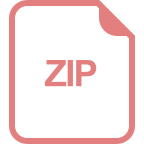














