用python写一个AI换脸代码
时间: 2023-12-17 11:43:50 浏览: 44
好的,下面是一个简单的用Python实现AI换脸的代码示例,仅供参考:
```python
import cv2
import dlib
import numpy as np
from imutils import face_utils
import argparse
def main():
# 解析命令行参数
ap = argparse.ArgumentParser()
ap.add_argument("-i", "--image", required=True, help="path to input image")
ap.add_argument("-m", "--model", default="./shape_predictor_68_face_landmarks.dat", help="path to facial landmark predictor")
args = vars(ap.parse_args())
# 加载图像并进行灰度化
image = cv2.imread(args["image"])
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 加载人脸检测器和关键点检测器
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor(args["model"])
# 检测人脸并提取关键点
rects = detector(gray, 1)
for (i, rect) in enumerate(rects):
shape = predictor(gray, rect)
shape = face_utils.shape_to_np(shape)
# 提取左眼、右眼、嘴巴和鼻子的关键点
left_eye = shape[42:48]
right_eye = shape[36:42]
mouth = shape[48:68]
nose = shape[27:36]
# 计算每个关键点的平均值
left_eye_center = np.mean(left_eye, axis=0).astype("int")
right_eye_center = np.mean(right_eye, axis=0).astype("int")
mouth_center = np.mean(mouth, axis=0).astype("int")
nose_center = np.mean(nose, axis=0).astype("int")
# 加载换脸图像
swap_img = cv2.imread("swap_image.jpg")
swap_img = cv2.resize(swap_img, (rect.width(), rect.height()))
# 提取换脸图像的关键点
swap_gray = cv2.cvtColor(swap_img, cv2.COLOR_BGR2GRAY)
swap_rects = detector(swap_gray, 1)
swap_shape = predictor(swap_gray, swap_rects[0])
swap_shape = face_utils.shape_to_np(swap_shape)
# 对换脸图像进行仿射变换,使其与当前图像的眼睛、嘴巴和鼻子位置对齐
M_left_eye = cv2.getAffineTransform(swap_shape[36:42], left_eye)
M_right_eye = cv2.getAffineTransform(swap_shape[42:48], right_eye)
M_mouth = cv2.getAffineTransform(swap_shape[48:68], mouth)
M_nose = cv2.getAffineTransform(swap_shape[27:36], nose)
swap_img = cv2.warpAffine(swap_img, M_left_eye, (image.shape[1], image.shape[0]))
swap_img = cv2.warpAffine(swap_img, M_right_eye, (image.shape[1], image.shape[0]))
swap_img = cv2.warpAffine(swap_img, M_mouth, (image.shape[1], image.shape[0]))
swap_img = cv2.warpAffine(swap_img, M_nose, (image.shape[1], image.shape[0]))
# 将换脸图像与当前图像进行融合
swap_mask = np.zeros(swap_gray.shape, dtype=np.uint8)
swap_mask = cv2.fillConvexPoly(swap_mask, cv2.convexHull(swap_shape), (255, 255, 255))
swap_mask = cv2.bitwise_not(swap_mask)
img_mask = np.zeros(image.shape, dtype=np.uint8)
img_mask[rect.top():rect.bottom(), rect.left():rect.right()] = swap_mask
swap_mask = cv2.bitwise_not(swap_mask)
swap_mask = np.stack((swap_mask,) * 3, axis=-1)
img = cv2.bitwise_and(image, swap_mask)
swap = cv2.bitwise_and(swap_img, img_mask)
result = cv2.add(img, swap)
# 显示结果
cv2.imshow("result", result)
cv2.waitKey(0)
if __name__ == '__main__':
main()
```
需要注意的是,这只是一个简单的示例,具体的实现方式和效果可能与你期望的有所不同。如果你需要更高质量的换脸效果,建议使用已有的成熟工具或者更深入的研究。
相关推荐
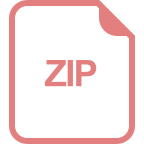
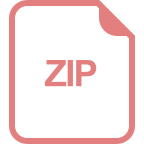
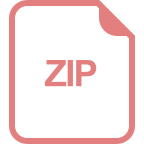














