#include<iostream> using namespace std; class Sample{ public: Sample(int i, int j) {x=i; y=j; } void disp() {cout<<"disp1"<<endl; } void disp() const } private: int x, y; }; int main() {const Sample a(1, 2); a. disp(); return 0; }
时间: 2024-01-03 16:03:13 浏览: 67
这段代码有一些语法错误。首先,在`void disp() const`之后应该有一个分号;其次,在`void disp() const`函数体内应该输出`disp2`而不是`disp1`,因为`const`成员函数不能修改对象的成员变量,所以不能调用非`const`的`disp()`函数。
以下是修改后的代码:
```c++
#include<iostream>
using namespace std;
class Sample {
public:
Sample(int i, int j) { x = i; y = j; }
void disp() { cout << "disp1" << endl; }
void disp() const { cout << "disp2" << endl; }
private:
int x, y;
};
int main() {
const Sample a(1, 2);
a.disp();
return 0;
}
```
输出结果为:
```
disp2
```
相关问题
#define _CRT_SECURE_NO_WARNINGS 1 #include<iostream> #include<cstring> using namespace std; class Sample { static int x; int y; char name[20]; public: Sample(int b, char* n) { y = b; strcpy(name, n); } }; int Sample::x = 0; int main() { Sample s1(10, "wang"), s2(20, "li"); cout << sizeof(s1) << sizeof(s2) << endl; return 0; }为什么在vs2022运行不了
这段代码存在一个问题:类中声明了静态成员变量x,但没有提供任何方法来初始化它。在C++中,静态成员变量必须在类外进行定义和初始化。因此,你需要在类外部添加一行代码来进行初始化:
int Sample::x = 0;
同时,你的代码中有一个编译警告,提示你应该使用 strncpy() 替代 strcpy() 来避免内存溢出问题。可以将 strcpy(name, n) 改为 strncpy(name, n, sizeof(name)-1)。
修改后的代码如下所示:
```
#define _CRT_SECURE_NO_WARNINGS 1
#include<iostream>
#include<cstring>
using namespace std;
class Sample {
static int x;
int y;
char name[20];
public:
Sample(int b, char* n) {
y = b;
strncpy(name, n, sizeof(name)-1);
}
};
int Sample::x = 0;
int main() {
Sample s1(10, "wang"), s2(20, "li");
cout << sizeof(s1) << sizeof(s2) << endl;
return 0;
}
```
#include <iostream> using namespace std; class Sample { public: int v; // 在此处补充你的代码 }; void PrintAndDouble(Sample o) { cout << o.v; cout << endl; } int main() { Sample a(5); Sample b = a; PrintAndDouble(b); Sample c = 20; PrintAndDouble(c); Sample d; d = a; cout << d.v; return 0; }
这段代码中,需要在 Sample 类中添加构造函数,以便能够用不同的方式初始化 Sample 类的对象。比如,可以添加无参构造函数、带参构造函数等。此外,还需要添加拷贝构造函数和拷贝赋值运算符,以便支持对象的复制和赋值操作。修改后的代码如下:
```
#include <iostream>
using namespace std;
class Sample {
public:
int v;
Sample():v(0){} // 无参构造函数
Sample(int x):v(x){} // 带参构造函数
Sample(const Sample& other){ // 拷贝构造函数
v = other.v;
}
Sample& operator=(const Sample& other){ // 拷贝赋值运算符
if(this != &other){
v = other.v;
}
return *this;
}
};
void PrintAndDouble(Sample o) {
cout << o.v;
cout << endl;
}
int main() {
Sample a(5);
Sample b = a;
PrintAndDouble(b);
Sample c = 20;
PrintAndDouble(c);
Sample d;
d = a;
cout << d.v;
return 0;
}
```
注意,这段代码中的 Sample 类中只定义了一个 int 类型的成员变量 v,因此在构造函数和拷贝函数中只需要对 v 进行赋值即可。如果 Sample 类中还有其他类型的成员变量,则需要在相应的构造函数和拷贝函数中对这些成员变量进行初始化或复制。
相关推荐
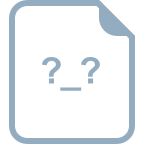













