Description 声明一个Shape抽象类,在此基础上派生出Rectangle类和Circle类,两者都有GetArea()和GetPerim()函数,用来计算面积和周长,麻烦封装以上各类,编写程序。需要提交类shape、Rectangle、Cricle类 Input 第一行输入n,表示n个测试例子;接下来有n行,每一行输入数,a、b、c,表示矩形的宽、长,以及圆形的半径 Output 对应每一行输入: 第一行输出矩形的面积、周长 第二行输出圆形的面积、周长 Sample_Input 1 2 2 3 Sample_Ouput 4 8 28.26 18.84 4 8 28.26 18.84 cpp:#include <iostream> using namespace std; const double PI = 3.14; #include "source.h" int main() { //freopen("in.txt", "r", stdin); //freopen("out.txt", "w", stdout); double n,a,b,c; cin>>n; for(int i=0;i<n;i++) { Shape *s; cin>>a>>b>>c; s = new Rectangle(a,b); cout<<s->GetArea()<<" "<<s->GetPerim()<<endl; delete s; s = new Circle(c); cout<<s->GetArea()<<" "<<s->GetPerim()<<endl; delete s; } return 0; }
时间: 2024-01-11 13:02:00 浏览: 55
shape.h:
```cpp
#ifndef SHAPE_H
#define SHAPE_H
class Shape {
public:
virtual double GetArea() = 0;
virtual double GetPerim() = 0;
};
#endif
```
rectangle.h:
```cpp
#ifndef RECTANGLE_H
#define RECTANGLE_H
#include "shape.h"
class Rectangle : public Shape {
public:
Rectangle(double width, double length);
virtual double GetArea();
virtual double GetPerim();
private:
double m_width;
double m_length;
};
#endif
```
rectangle.cpp:
```cpp
#include "rectangle.h"
Rectangle::Rectangle(double width, double length)
: m_width(width), m_length(length) {}
double Rectangle::GetArea() {
return m_width * m_length;
}
double Rectangle::GetPerim() {
return 2 * (m_width + m_length);
}
```
circle.h:
```cpp
#ifndef CIRCLE_H
#define CIRCLE_H
#include "shape.h"
class Circle : public Shape {
public:
Circle(double radius);
virtual double GetArea();
virtual double GetPerim();
private:
double m_radius;
};
#endif
```
circle.cpp:
```cpp
#include "circle.h"
#include <cmath>
Circle::Circle(double radius)
: m_radius(radius) {}
double Circle::GetArea() {
return PI * pow(m_radius, 2);
}
double Circle::GetPerim() {
return 2 * PI * m_radius;
}
```
相关推荐
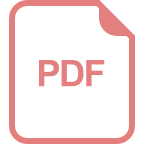
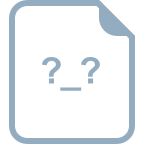
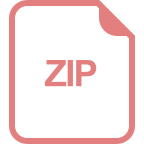















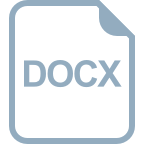