self.graphicsView.addItem(self.path) ^^^^^^^^^^^^^^^^^^^^^^^^^ AttributeError: 'QGraphicsView' object has no attribute 'addItem'
时间: 2024-11-06 22:12:43 浏览: 13
这个`AttributeError`提示你试图在一个`QGraphicsView`对象上调用`addItem`方法,但实际上`QGraphicsView`本身并不直接支持这个操作。`QGraphicsView`是一个视图组件,它用来显示`QGraphicsScene`的内容,而不是直接参与到`QGraphicsScene`的管理中去。
你应该在关联的`QGraphicsScene`上使用`addItem`方法,因为`QGraphicsView`内部有一个`QGraphicsScene`对象,你可以通过它的`scene()`属性访问。所以正确的代码应该是这样的:
```python
scene = self.graphicsView.scene() # 获取QGraphicsScene对象
scene.addItem(self.path) # 在scene上添加item
```
如果`graphicsView`尚未关联到场景,你需要先设置`QGraphicsView`的`scene`属性:
```python
if not self.graphicsView.scene():
self.graphicsView.setScene(scene) # 设置QGraphicsView的场景
```
相关问题
每次按键按下后self.graphicsView = QtWidgets.QGraphicsView(self.centralwidget) self.graphicsView.setGeometry(QtCore.QRect(20, 200, 621, 361)) self.graphicsView.setObjectName("graphicsView")更新显示以时间命名的图片
可以使用PyQt5中的QPixmap和QGraphicsPixmapItem类来实现在QGraphicsView中显示图片的功能。具体的代码如下:
```python
from PyQt5.QtGui import QPixmap
from PyQt5.QtWidgets import QGraphicsPixmapItem
# 添加新的图片到新的文件夹中
# 每次按下按键后执行以下代码
new_image_name = time.strftime("%Y%m%d%H%M%S", time.localtime()) + ".jpg" # 新图片名称,使用当前时间来命名
new_image_path = os.path.join(new_folder_name, new_image_name) # 新图片路径
# 将新的图片保存到新的文件夹中
# ...
pixmap = QPixmap(new_image_path) # 创建一个QPixmap对象,用来显示图片
pixmap_item = QGraphicsPixmapItem(pixmap) # 创建一个QGraphicsPixmapItem对象,用来在QGraphicsView中显示图片
self.graphicsView.setScene(self.scene) # 将QGraphicsView的场景设置为self.scene
self.scene.addItem(pixmap_item) # 在self.scene中添加新的QGraphicsPixmapItem对象,用来显示图片
```
上述代码将新的图片加载到QPixmap对象中,并创建一个QGraphicsPixmapItem对象用来在QGraphicsView中显示图片。然后将QGraphicsView的场景设置为self.scene,再在self.scene中添加新的QGraphicsPixmapItem对象,用来显示图片。这样就可以实现每次按下按键后在QGraphicsView中更新显示以时间命名的图片。
pyqt5 graphicsView 加载显示图片,在图片上鼠标框选红色矩形并返回矩形在图片上的坐标
可以通过以下步骤实现:
1. 创建一个 GraphicsView 对象和一个 QGraphicsScene 对象。
```python
from PyQt5.QtWidgets import QGraphicsView, QGraphicsScene
view = QGraphicsView()
scene = QGraphicsScene()
view.setScene(scene)
```
2. 在 QGraphicsScene 中添加图片。
```python
from PyQt5.QtGui import QPixmap
pixmap = QPixmap("path/to/image.jpg")
scene.addPixmap(pixmap)
```
3. 创建一个 QGraphicsRectItem 对象,用于表示红色矩形。
```python
from PyQt5.QtGui import QPen, QColor
from PyQt5.QtCore import Qt, QRectF
from PyQt5.QtWidgets import QGraphicsRectItem
pen = QPen(QColor(Qt.red))
pen.setWidth(2)
rect_item = QGraphicsRectItem()
rect_item.setPen(pen)
```
4. 在 GraphicsView 中重载 mousePressEvent 和 mouseReleaseEvent 方法,分别记录鼠标点击和释放的位置。
```python
class MyGraphicsView(QGraphicsView):
def __init__(self):
super().__init__()
def mousePressEvent(self, event):
self.start_pos = event.pos()
def mouseReleaseEvent(self, event):
end_pos = event.pos()
self.draw_rect(self.start_pos, end_pos)
def draw_rect(self, start_pos, end_pos):
# 计算矩形的位置和大小
rect = QRectF(start_pos, end_pos)
rect = rect.normalized()
# 设置矩形的位置和大小
rect_item.setRect(rect)
# 将矩形添加到场景中
scene.addItem(rect_item)
# 计算矩形在图片上的位置
rect_in_image = view.mapToScene(rect_item.boundingRect()).boundingRect()
print(rect_in_image)
```
5. 在 draw_rect 方法中,计算矩形在图片上的位置,并输出结果。
完整代码如下:
```python
from PyQt5.QtWidgets import QGraphicsView, QGraphicsScene
from PyQt5.QtGui import QPixmap, QPen, QColor
from PyQt5.QtCore import Qt, QRectF
view = QGraphicsView()
scene = QGraphicsScene()
view.setScene(scene)
pixmap = QPixmap("path/to/image.jpg")
scene.addPixmap(pixmap)
pen = QPen(QColor(Qt.red))
pen.setWidth(2)
rect_item = QGraphicsRectItem()
rect_item.setPen(pen)
class MyGraphicsView(QGraphicsView):
def __init__(self):
super().__init__()
def mousePressEvent(self, event):
self.start_pos = event.pos()
def mouseReleaseEvent(self, event):
end_pos = event.pos()
self.draw_rect(self.start_pos, end_pos)
def draw_rect(self, start_pos, end_pos):
rect = QRectF(start_pos, end_pos)
rect = rect.normalized()
rect_item.setRect(rect)
scene.addItem(rect_item)
rect_in_image = view.mapToScene(rect_item.boundingRect()).boundingRect()
print(rect_in_image)
view = MyGraphicsView()
view.show()
```
阅读全文
相关推荐
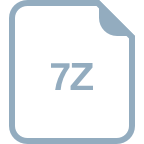
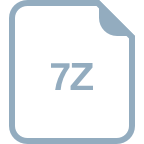
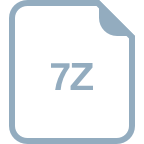



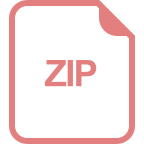
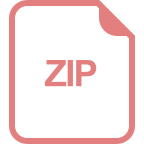
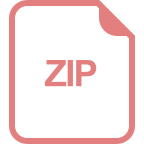
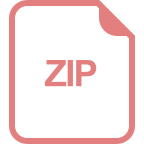
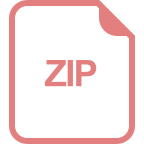
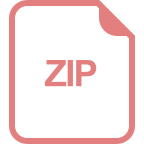
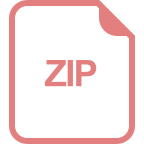
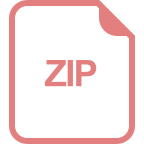