#include <stdio.h> #include <stdlib.h> #include <string.h> #include <stdint.h> typedef struct { char *key; char *value; } KeyValuePair; typedef struct { uint32_t start_address; uint32_t block_size; uint16_t checksum; uint32_t block_start; } DataBlock; typedef struct { KeyValuePair* header_KeyValuePair; DataBlock* blocklist; } VBFInfo; VBFInfo *GetVBFinfo(char *vbfpath) { FILE *fp; fp = fopen(vbfpath, "rb"); if(fp == NULL) { printf("Error opening file."); exit(1); } /* Read file header */ char *header; int aaa; while(1) { char byte; fread(&byte, 1, 1, fp); header[strlen(header)] = byte; if(byte == '{') { aaa= 1; break; } } fseek(fp, ftell(fp), SEEK_SET); //文件指针定位 if(aaa > 0) { while(1) { char byte; fread(&byte, 1, 1, fp); header[strlen(header)] = byte; if(byte == '{') { aaa++; } if(byte == '}') { aaa--; if(aaa == 0) { break; } } } } //截取header信息 char delimiter[] = " =\";\n"; char* token = strtok(header, delimiter); int i = 0; while (token != NULL) { if (i % 2 == 0) { // 键名在偶数位置 strcpy(key_value_list[i / 2].key, token); } else { // 键值在奇数位置 strcpy(key_value_list[i / 2].value, token); } token = strtok(NULL, delimiter); i++; } if (i % 2 != 0) { // 最后一个键名没有对应的键值 *num_entries = i / 2 - 1; } else { *num_entries = i / 2; } }
时间: 2024-03-17 20:39:46 浏览: 74
这段代码存在多处问题,可能会导致编译错误或运行时错误。下面是一些潜在问题:
1. 在代码的开头没有定义变量 `key_value_list` 和 `num_entries`,这两个变量在后面的代码中被使用了。你需要在代码的开头定义它们,例如 `KeyValuePair key_value_list[100]; int num_entries = 0;`。
2. 变量 `header` 没有被初始化,当你尝试将一个字符添加到它的末尾时,会引发未定义的行为。你需要在使用 `header` 变量之前为其分配足够的内存空间,例如 `char header[1000];`。
3. 当读取文件头时,你将每个字节都添加到 `header` 变量的末尾。但是在第一次循环时,`header` 是一个未初始化的变量,因此 `header[strlen(header)]` 的结果是未定义的。你需要在循环之前为 `header` 变量分配足够的内存空间,并将其初始化为空字符串。
4. 在代码的最后,你没有返回任何值。由于函数的返回类型是 `VBFInfo*`,你需要在函数的结尾处返回一个指向 `VBFInfo` 结构体的指针。
5. 在代码中没有检查 `header` 变量的长度是否超出了它的缓冲区大小。如果文件头的长度超过了 `header` 变量的大小,那么可能会发生缓冲区溢出,导致未定义的行为。你应该在读取文件头时检查 `header` 变量的长度,并确保它不会超出缓冲区大小。
6. 变量 `key_value_list` 和 `num_entries` 没有作为参数传递给函数 `GetVBFinfo`,因此在函数中无法访问它们。你需要将它们作为参数传递给函数,例如 `VBFInfo *GetVBFinfo(char *vbfpath, KeyValuePair key_value_list[], int *num_entries)`。
7. 在函数中没有为结构体 `VBFInfo` 分配内存空间。你需要在函数开始时为 `VBFInfo` 结构体分配内存空间,例如 `VBFInfo *vbf_info = (VBFInfo*)malloc(sizeof(VBFInfo));`。
修复了上述问题后,代码可能如下所示:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdint.h>
typedef struct {
char *key;
char *value;
} KeyValuePair;
typedef struct {
uint32_t start_address;
uint32_t block_size;
uint16_t checksum;
uint32_t block_start;
} DataBlock;
typedef struct {
KeyValuePair* header_KeyValuePair;
DataBlock* blocklist;
} VBFInfo;
VBFInfo *GetVBFinfo(char *vbfpath, KeyValuePair key_value_list[], int *num_entries) {
FILE *fp;
fp = fopen(vbfpath, "rb");
if(fp == NULL) {
printf("Error opening file.");
exit(1);
}
/* Read file header */
char header[1000] = "";
int aaa = 0;
while(1) {
char byte;
fread(&byte, 1, 1, fp);
if(strlen(header) < sizeof(header) - 1) {
header[strlen(header)] = byte;
}
if(byte == '{') {
aaa = 1;
break;
}
}
fseek(fp, ftell(fp), SEEK_SET); //文件指针定位
if(aaa > 0) {
while(1) {
char byte;
fread(&byte, 1, 1, fp);
if(strlen(header) < sizeof(header) - 1) {
header[strlen(header)] = byte;
}
if(byte == '{') {
aaa++;
}
if(byte == '}') {
aaa--;
if(aaa == 0) {
break;
}
}
}
}
/* Parse header information */
char delimiter[] = " =\";\n";
char* token = strtok(header, delimiter);
int i = 0;
while (token != NULL) {
if (i % 2 == 0) { // 键名在偶数位置
strcpy(key_value_list[i / 2].key, token);
} else { // 键值在奇数位置
strcpy(key_value_list[i / 2].value, token);
}
token = strtok(NULL, delimiter);
i++;
}
if (i % 2 != 0) { // 最后一个键名没有对应的键值
*num_entries = i / 2 - 1;
} else {
*num_entries = i / 2;
}
/* Allocate memory for VBFInfo struct */
VBFInfo *vbf_info = (VBFInfo*)malloc(sizeof(VBFInfo));
vbf_info->header_KeyValuePair = key_value_list;
vbf_info->blocklist = NULL;
return vbf_info;
}
```
阅读全文
相关推荐
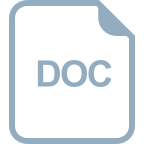
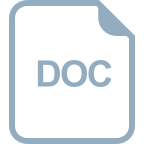
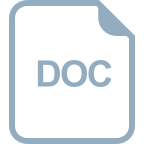















