生成对抗网络时间序列预测代码
时间: 2023-08-06 10:22:27 浏览: 59
生成对抗网络(GAN)也可以用于时间序列预测。以下是一个简单的GAN时间序列预测代码示例:
```python
import torch
import torch.nn as nn
import torch.optim as optim
import numpy as np
import matplotlib.pyplot as plt
# 定义生成器
class Generator(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super(Generator, self).__init__()
self.fc1 = nn.Linear(input_size, hidden_size)
self.fc2 = nn.Linear(hidden_size, hidden_size)
self.fc3 = nn.Linear(hidden_size, output_size)
self.relu = nn.ReLU()
self.tanh = nn.Tanh()
def forward(self, x):
x = self.relu(self.fc1(x))
x = self.relu(self.fc2(x))
x = self.tanh(self.fc3(x))
return x
# 定义判别器
class Discriminator(nn.Module):
def __init__(self, input_size, hidden_size, output_size):
super(Discriminator, self).__init__()
self.fc1 = nn.Linear(input_size, hidden_size)
self.fc2 = nn.Linear(hidden_size, hidden_size)
self.fc3 = nn.Linear(hidden_size, output_size)
self.relu = nn.ReLU()
self.sigmoid = nn.Sigmoid()
def forward(self, x):
x = self.relu(self.fc1(x))
x = self.relu(self.fc2(x))
x = self.sigmoid(self.fc3(x))
return x
# 定义超参数
input_size = 10
hidden_size = 32
output_size = 1
batch_size = 64
num_epochs = 500
lr = 0.0002
# 生成数据
def generate_data():
x = np.linspace(0, 10, 1000)
y = np.sin(x) + np.random.normal(0, 0.1, 1000)
return x, y
x, y = generate_data()
# 数据预处理
def preprocess_data(x, y, input_size):
data = []
for i in range(len(x) - input_size):
data.append(y[i:i+input_size])
data = np.array(data)
data = (data - np.min(data)) / (np.max(data) - np.min(data))
return data
data = preprocess_data(x, y, input_size)
# 转换为张量
data = torch.FloatTensor(data)
# 初始化生成器和判别器
G = Generator(input_size, hidden_size, output_size)
D = Discriminator(input_size, hidden_size, output_size)
# 定义损失函数和优化器
criterion = nn.BCELoss()
G_optimizer = optim.Adam(G.parameters(), lr=lr)
D_optimizer = optim.Adam(D.parameters(), lr=lr)
# 训练模型
for epoch in range(num_epochs):
for i in range(len(data) // batch_size):
# 训练判别器
D.zero_grad()
real_labels = torch.ones(batch_size, 1)
fake_labels = torch.zeros(batch_size, 1)
real_data = data[i*batch_size:(i+1)*batch_size]
real_outputs = D(real_data)
real_loss = criterion(real_outputs, real_labels)
z = torch.randn(batch_size, input_size)
fake_data = G(z)
fake_outputs = D(fake_data)
fake_loss = criterion(fake_outputs, fake_labels)
D_loss = real_loss + fake_loss
D_loss.backward()
D_optimizer.step()
# 训练生成器
G.zero_grad()
z = torch.randn(batch_size, input_size)
fake_data = G(z)
fake_outputs = D(fake_data)
G_loss = criterion(fake_outputs, real_labels)
G_loss.backward()
G_optimizer.step()
# 打印损失值
if epoch % 50 == 0:
print('Epoch [{}/{}], D Loss: {:.4f}, G Loss: {:.4f}'
.format(epoch, num_epochs, D_loss.item(), G_loss.item()))
# 生成预测数据
z = torch.randn(len(data) - input_size, input_size)
pred_data = G(z).detach().numpy()
pred_data = np.concatenate((np.zeros((input_size, 1)), pred_data), axis=0)
pred_data = pred_data * (np.max(data) - np.min(data)) + np.min(data)
# 绘制结果
plt.plot(x, y, label='ground truth')
plt.plot(x, pred_data, label='prediction')
plt.legend()
plt.show()
```
在这个例子中,我们使用了PyTorch框架来实现GAN时间序列预测。首先定义了生成器和判别器的神经网络结构,然后定义了超参数和生成数据的函数。接着,我们对数据进行预处理,转换为张量,并迭代训练模型,其中每个迭代步骤都包括训练判别器和生成器。最后,我们生成预测数据并绘制结果。
相关推荐
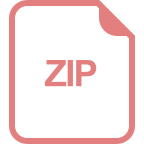
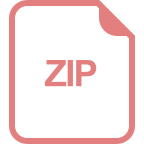
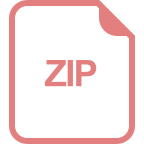














