用c++代码实现操作系统rr调度算法,要求:1)输入时间片和各进程运行时间(以空格间隔),手动输入。 2)结果输出各进程的开始时间和结束时间、进程的平均等待时间,和具体的甘特图。
时间: 2024-03-10 10:45:36 浏览: 95
以下是一个C++代码示例,实现了Round Robin调度算法并输出相关结果:
```c++
#include <iostream>
#include <queue>
#include <vector>
using namespace std;
// 定义进程结构体
struct Process {
int id; // 进程ID
int arrival; // 到达时间
int burst; // 执行时间
int remaining; // 剩余执行时间
};
// 定义进程比较函数,按到达时间升序排列
struct ProcessCompare {
bool operator()(const Process& p1, const Process& p2) {
return p1.arrival > p2.arrival;
}
};
int main() {
int n; // 进程数量
int quantum; // 时间片大小
int total_time = 0; // 总执行时间
double total_wait_time = 0; // 总等待时间
priority_queue<Process, vector<Process>, ProcessCompare> arrival_queue; // 到达队列
queue<Process> ready_queue; // 就绪队列
// 手动输入进程信息
cout << "Enter the number of processes: ";
cin >> n;
cout << "Enter the time quantum: ";
cin >> quantum;
for (int i = 0; i < n; i++) {
int arrival, burst;
cout << "Enter the arrival time and burst time of process " << i + 1 << ": ";
cin >> arrival >> burst;
Process p = {i + 1, arrival, burst, burst};
arrival_queue.push(p);
total_time += burst;
}
// 执行调度
int current_time = 0;
vector<pair<int, int>> gantt_chart; // 甘特图
while (current_time < total_time) {
// 处理到达队列中到达时间小于等于当前时间的进程
while (!arrival_queue.empty() && arrival_queue.top().arrival <= current_time) {
ready_queue.push(arrival_queue.top());
arrival_queue.pop();
}
// 处理就绪队列中的进程
if (!ready_queue.empty()) {
Process p = ready_queue.front();
ready_queue.pop();
int start_time = current_time;
if (p.remaining > quantum) {
// 如果剩余执行时间大于时间片大小,执行一次时间片
current_time += quantum;
p.remaining -= quantum;
// 将进程重新加入就绪队列
ready_queue.push(p);
} else {
// 如果剩余执行时间小于等于时间片大小,执行完当前进程
current_time += p.remaining;
p.remaining = 0;
total_wait_time += current_time - p.arrival - p.burst;
cout << "Process " << p.id << ": " << start_time << "-" << current_time << endl;
}
gantt_chart.push_back(make_pair(start_time, current_time));
} else {
// 如果就绪队列为空,时间直接跳到下一个进程到达时间
current_time = arrival_queue.top().arrival;
}
}
// 输出结果
cout << "Average wait time: " << total_wait_time / n << endl;
cout << "Gantt chart:" << endl;
for (auto p : gantt_chart) {
cout << p.first << "-" << p.second << " | ";
}
cout << endl;
return 0;
}
```
这段代码手动输入进程信息,包括进程数量、时间片大小和每个进程的到达时间和执行时间,然后执行Round Robin调度算法。在执行调度时,先处理到达队列中到达时间小于等于当前时间的进程,将它们加入就绪队列;然后从就绪队列中取出一个进程,如果它的剩余执行时间大于时间片大小,则执行一次时间片并将其重新加入就绪队列,否则执行完当前进程。在处理完一个进程后,记录它的开始时间和结束时间,并将它的等待时间累加到总等待时间中。最终输出各进程的开始时间和结束时间、进程的平均等待时间,以及具体的甘特图。
阅读全文
相关推荐
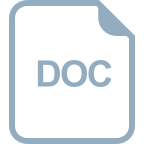
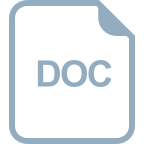
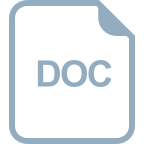
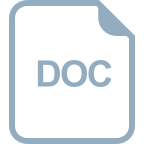
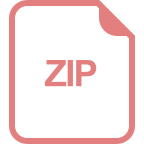
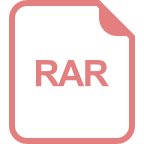
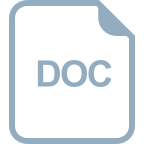
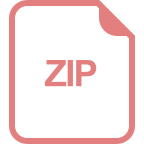
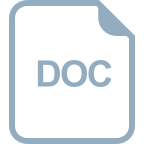
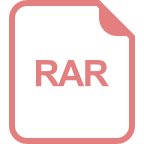
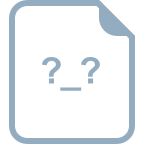
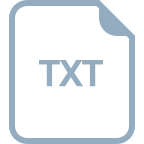
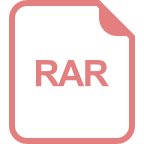
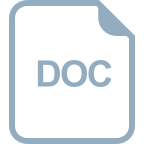
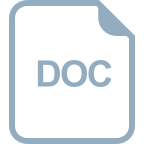
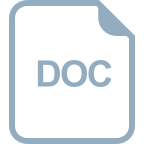
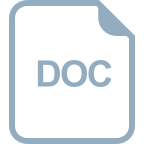
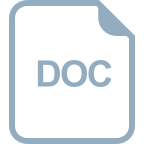