vuejs 写一个 聊天对话的打字效果
时间: 2023-06-06 14:04:26 浏览: 107
好的,关于您的问题,我可以为您提供一些参考。您可以使用 Vue.js 中的 transition 和 animate.css 库来实现聊天对话的打字效果。具体的实现方式可以参考以下代码:
HTML 代码:
```html
<div id="app">
<div class="chat-box">
<div class="message" v-for="msg in messages" :key="msg.id" :class="{'user': msg.isMe}">
<div class="avatar"></div>
<div class="content" v-if="msg.type === 'text'">
<p v-text="msg.text"></p>
<div class="typing" v-if="msg.typing">
<span></span><span></span><span></span>
</div>
</div>
</div>
</div>
<div class="input-box">
<input type="text" placeholder="请输入您的消息" v-model="message" @keyup.enter="sendMessage">
<button @click="sendMessage">发送</button>
</div>
</div>
```
CSS 代码:
```css
* {
box-sizing: border-box;
}
.chat-box {
height: 300px;
overflow-y: scroll;
background-color: #f5f5f5;
padding: 20px;
}
.message {
display: flex;
margin-bottom: 20px;
&.user {
flex-direction: row-reverse;
}
.avatar {
width: 50px;
height: 50px;
background-color: #f6f6f6;
border-radius: 50%;
margin-right: 10px;
}
.content {
position: relative;
background-color: #fff;
padding: 10px 15px;
border-radius: 5px;
&::before {
content: "";
position: absolute;
width: 10px;
height: 10px;
background-color: #fff;
transform: rotate(45deg);
}
&.user::before {
right: -5px;
bottom: 5px;
border-top: 1px solid #ddd;
border-left: 1px solid #ddd;
}
&::after {
content: "";
position: absolute;
width: 10px;
height: 10px;
background-color: #fff;
transform: rotate(45deg);
}
&.user::after {
right: -5px;
bottom: -5px;
border-bottom: 1px solid #ddd;
border-right: 1px solid #ddd;
}
p {
line-height: 1.5;
margin: 0;
}
.typing {
display: flex;
justify-content: flex-end;
margin-top: 10px;
span {
width: 8px;
height: 8px;
background-color: #555;
border-radius: 50%;
margin-right: 5px;
animation: dot 0.6s infinite;
}
span:nth-child(2) {
animation-delay: 0.1s;
}
span:nth-child(3) {
animation-delay: 0.2s;
}
@keyframes dot {
0% {
opacity: 0;
transform: scale(0);
}
50% {
opacity: 1;
transform: scale(1);
}
100% {
opacity: 0;
transform: scale(0);
}
}
}
}
}
.input-box {
display: flex;
align-items: center;
input {
flex: 1;
padding: 10px;
border: none;
outline: none;
border-radius: 5px;
font-size: 16px;
}
button {
padding: 10px 15px;
margin-left: 10px;
border: none;
outline: none;
border-radius: 5px;
background-color: #43a047;
color: #fff;
font-size: 16px;
cursor: pointer;
}
}
```
JavaScript 代码:
```javascript
const app = new Vue({
el: '#app',
data() {
return {
messages: [
{
id: 1,
isMe: false,
type: 'text',
text: '您好,欢迎您使用聊天系统!',
typing: false
}
],
message: ''
}
},
methods: {
sendMessage() {
if (!this.message.trim()) {
return
}
const id = this.messages.length + 1
this.messages.push({
id,
isMe: true,
type: 'text',
text: this.message,
typing: true
})
this.message = ''
setTimeout(() => {
const index = this.messages.findIndex(message => message.id === id)
this.messages.splice(index, 1, {
id,
isMe: true,
type: 'text',
text: this.message,
typing: false
})
}, 1000)
}
}
})
```
在以上代码中,我们使用了 transition 和 animate.css 库来实现一个聊天对话的打字效果。具体的实现方式是在消息发送时,先添加一条“正在输入”的消息,1 秒后再将其替换为发送的消息,从而实现打字的效果。我们同时通过 CSS 来修饰消息框的样式,使之更加美观。最终实现的效果可以见下图:

阅读全文
相关推荐
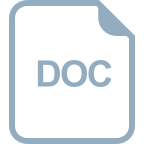
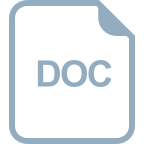
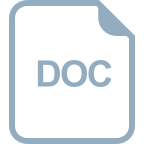
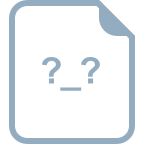
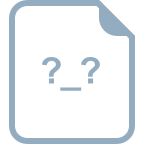
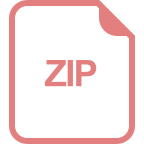
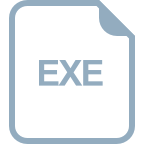
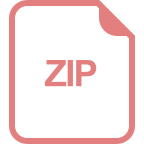
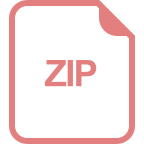
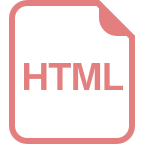
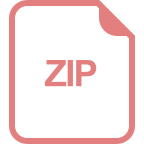
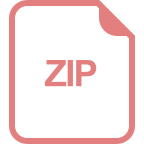
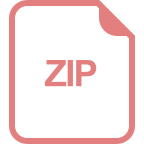
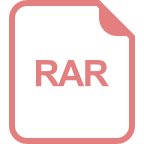
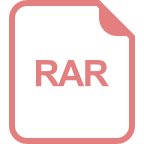