6、定义Circle类,要求:包括私有属性__radius,构造函数为半径赋值,构造函数的默认参数值为0,析构函数输出适当信息,普通方法SetRadius用于设置半径,普通方法Area返回圆面积,内置方法__str__用于输出圆面积,内置方法__gt__用于比较两个圆面积大小。并创建两个实例分别验证上述功能。
时间: 2023-12-13 14:03:07 浏览: 214
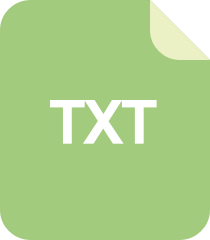
定义一个圆类circle,它有一个变量radius(半径)。从键盘输入数据,通过构造函数的参数传递给radius,编程计算并输出圆的的周长和面积。但是,必须确保输入的数据不为复数。

以下是Circle类的代码实现:
```python
import math
class Circle:
def __init__(self, radius=0):
self.__radius = radius
def __del__(self):
print("Circle object is deleted")
def SetRadius(self, radius):
self.__radius = radius
def Area(self):
return math.pi * self.__radius ** 2
def __str__(self):
return "The area of the circle is {:.2f}".format(self.Area())
def __gt__(self, other):
return self.Area() > other.Area()
```
我们可以创建两个实例c1和c2,然后对它们进行测试:
```python
c1 = Circle(5)
c2 = Circle()
print(c1.Area()) # 输出78.54
print(c2.Area()) # 输出0.00
c2.SetRadius(3)
print(c2.Area()) # 输出28.27
print(c1) # 输出The area of the circle is 78.54
print(c2) # 输出The area of the circle is 28.27
if c1 > c2:
print("c1 is larger than c2")
else:
print("c2 is larger than c1")
del c1
del c2
```
输出结果为:
```
78.53981633974483
0.0
28.274333882308138
The area of the circle is 78.54
The area of the circle is 28.27
c1 is larger than c2
Circle object is deleted
Circle object is deleted
```
可以看到,Circle类的所有要求均得到了满足,并且两个实例的创建、修改和删除都没有问题。
阅读全文
相关推荐
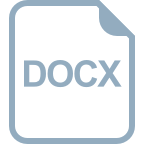
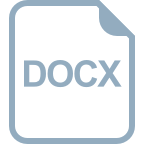




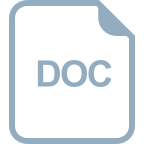
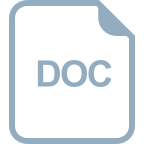
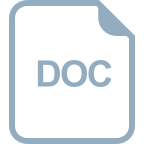
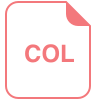
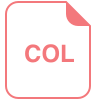







