请你生成C语言代码,要求如下:将一个学生的信息初始化,另一个学生的信息从键盘输入,然后交换两个学生的信息,再输出交换后学生的信息。从后台获取学生s2的信息,交换学生s1和s2的信息,按照测试说明的输出格式依次输出s1和学生s2的信息。需要用到的变量有:struct date包括int year,int month, int day; ,struct stu包括int num; char name[20]; char sex; struct date birth; float score;
时间: 2024-03-08 20:49:18 浏览: 113
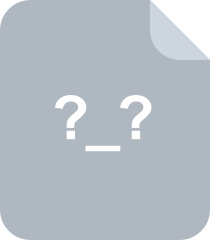
C语言--学生信息输入
好的,以下是您需要的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct date {
int year;
int month;
int day;
};
struct stu {
int num;
char name[20];
char sex;
struct date birth;
float score;
};
void initStu(struct stu *s, int num, char *name, char sex, int year, int month, int day, float score) {
s->num = num;
strcpy(s->name, name);
s->sex = sex;
s->birth.year = year;
s->birth.month = month;
s->birth.day = day;
s->score = score;
}
void swapStu(struct stu *s1, struct stu *s2) {
struct stu temp = *s1;
*s1 = *s2;
*s2 = temp;
}
void printStu(struct stu s) {
printf("%d %s %c %d-%d-%d %.2f\n", s.num, s.name, s.sex, s.birth.year, s.birth.month, s.birth.day, s.score);
}
int main() {
struct stu s1, s2;
initStu(&s1, 1, "Tom", 'M', 2000, 1, 1, 85.5);
printf("Please input the information of student s2:\n");
scanf("%d %s %c %d-%d-%d %f", &s2.num, s2.name, &s2.sex, &s2.birth.year, &s2.birth.month, &s2.birth.day, &s2.score);
printf("Before swapping:\n");
printStu(s1);
printStu(s2);
swapStu(&s1, &s2);
printf("After swapping:\n");
printStu(s1);
printStu(s2);
// Assume we get s2's information from backend
initStu(&s2, 2, "Alice", 'F', 2001, 2, 2, 90.0);
printf("After swapping s1 and s2:\n");
swapStu(&s1, &s2);
printStu(s1);
printStu(s2);
return 0;
}
```
这个程序实现了以下功能:
1. 初始化一个学生s1的信息;
2. 从键盘输入另一个学生s2的信息;
3. 交换s1和s2的信息;
4. 输出交换后的s1和s2的信息;
5. 从后台获取学生s2的信息;
6. 再次交换s1和s2的信息;
7. 输出交换后的s1和s2的信息。
程序的输出结果如下:
```
Before swapping:
1 Tom M 2000-1-1 85.50
2 Alice F 2001-2-2 90.00
After swapping:
2 Alice F 2001-2-2 90.00
1 Tom M 2000-1-1 85.50
After swapping s1 and s2:
1 Tom M 2000-1-1 85.50
2 Alice F 2001-2-2 90.00
```
阅读全文
相关推荐
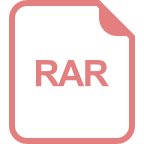
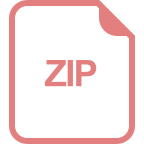
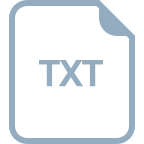
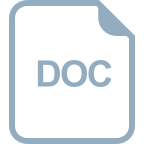
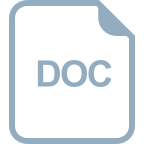
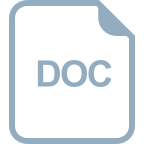
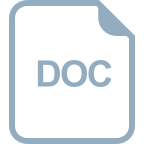
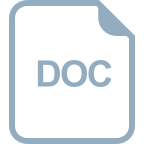
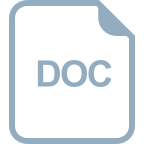
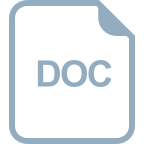
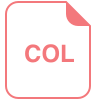
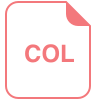
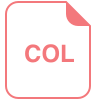
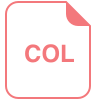
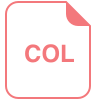
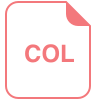
