在windows系统中,如何使用python创建一个虚拟摄像头,并且使用onvif协议进行发布给NVR
时间: 2024-11-18 11:26:58 浏览: 68
在Windows系统中,你可以使用Python结合一些库来创建虚拟摄像头并利用ONVIF协议与网络视频录像机(NVR)通信。这里推荐使用以下几个步骤和技术:
1. **安装必要的库**:
- OpenCV(cv2):用于图像处理和摄像头操作。
- Pyzbar:如果需要扫描条形码或二维码。
- onvifpy:一个Python ONVIF客户端库,用于连接到ONVIF兼容设备(包括NVR)。
```bash
pip install opencv-python numpy pyzbar onvifpy
```
2. **创建虚拟摄像头**:
使用OpenCV的VideoCapture对象模拟一个虚拟摄像头。例如,可以读取一个图片序列或者生成一个静态图像作为源:
```python
import cv2
# 创建一个空白的黑白帧
img = np.zeros((640, 480, 3), dtype=np.uint8)
cap = cv2.VideoCapture(-1) # Use -1 for the virtual camera
cap.set(cv2.CAP_PROP_FRAME_WIDTH, 640) # Set your desired frame width
cap.set(cv2.CAP_PROP_FRAME_HEIGHT, 480) # Set your desired frame height
while True:
ret, frame = cap.read()
if not ret:
break
# Process and display the frame
cv2.imshow('Virtual Camera', frame)
if cv2.waitKey(1) & 0xFF == ord('q'): # Quit on 'q' key press
break
cap.release()
cv2.destroyAllWindows()
```
3. **ONVIF连接和发布流**:
使用onvifpy库连接到NVR,并将摄像头流添加到设备的服务中:
```python
from onvif import ONVIFCamera
def create_onvif_device():
device_url = "http://your_nvr_ip:80/ONVIF/device_service" # Replace with NVR IP and port
username = "username"
password = "password"
camera = ONVIFCamera(device_url, username, password)
return camera.create_media_service()
def publish_stream(camera):
profile = camera.get_profile() # Get the first available profile
stream_config = profile.create_media_input_config("YourInputName") # Input name can be any unique string
stream_profile = profile.create_media_output_profile(stream_config)
stream_profile.publish("Virtual Camera Stream")
camera = create_onvif_device()
try:
publish_stream(camera)
except Exception as e:
print(f"Error publishing stream: {e}")
finally:
camera.close()
```
确保替换URL、用户名和密码为你实际NVR的配置。
阅读全文
相关推荐
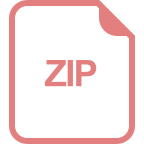
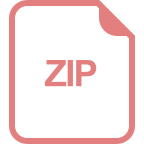

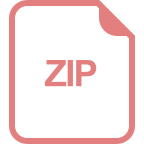
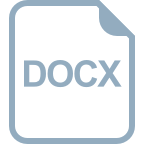
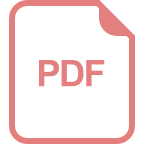
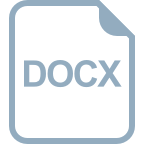
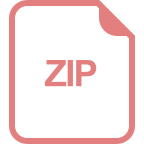
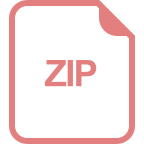
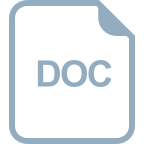
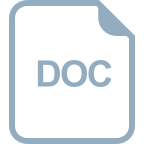
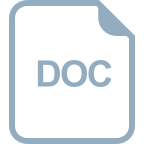
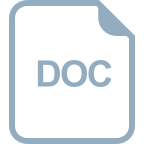
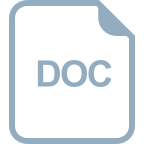
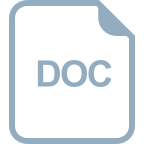

