vue限制input不能输入小数点,给出代码
时间: 2024-04-13 20:30:47 浏览: 114
当您使用 Vue 框架时,可以通过使用 `input` 事件和正则表达式来限制输入框不能输入小数点。下面是一个示例代码:
```html
<template>
<div>
<input type="text" v-model="inputValue" @input="handleInput" />
</div>
</template>
<script>
export default {
data() {
return {
inputValue: ""
};
},
methods: {
handleInput(event) {
// 使用正则表达式过滤输入值,不允许小数点
this.inputValue = event.target.value.replace(/\./g, "");
}
}
};
</script>
```
在上述代码中,我们使用 `v-model` 指令绑定输入框的值到 `inputValue` 属性上,并在 `@input` 事件中调用 `handleInput` 方法。在 `handleInput` 方法中,我们使用正则表达式 `/\.+/g` 过滤输入值,将所有小数点替换为空字符串。这样就可以限制输入框不能输入小数点。
请注意,这只是一个示例代码,您可以根据您的实际需求进行调整和修改。希望对您有所帮助!
相关问题
ant-desgin-vue表单input只能数字、小数点和保留两位小数
您可以使用 Ant Design Vue 表单组件中的 `<a-input>` 组件,并在其 `onInput` 事件中添加一个函数来限制输入只能是数字和小数点,并保留两位小数。以下是一个示例代码:
```vue
<template>
<a-form>
<a-form-item label="只能输入数字和小数点,保留两位小数">
<a-input v-model="inputValue" @input="handleInput" />
</a-form-item>
</a-form>
</template>
<script>
export default {
data() {
return {
inputValue: ''
}
},
methods: {
handleInput(e) {
let value = e.target.value
// 只允许输入数字和小数点
value = value.replace(/[^\d\.]/g, '')
// 只保留两位小数
const pointIndex = value.indexOf('.')
if (pointIndex !== -1 && value.length - pointIndex > 3) {
value = value.slice(0, pointIndex + 3)
}
this.inputValue = value
}
}
}
</script>
```
在上面的代码中,我们使用正则表达式 `[^\d\.]` 来匹配非数字和非小数点的字符,并将其替换为空字符串。然后,我们检查输入中是否包含小数点,并且小数点后面是否有超过两位数字。如果是,则将输入值截断为保留两位小数。最后,我们将处理后的值更新到 `inputValue` 变量中,以便在表单中显示。
ant-desgin-vue表单input只能数字、小数点、负号和保留两位小数
您可以使用 Ant Design Vue 表单组件中的 `<a-input>` 组件,并在其 `onInput` 事件中添加一个函数来限制输入只能是数字、小数点和负号,并保留两位小数。以下是一个示例代码:
```vue
<template>
<a-form>
<a-form-item label="只能输入数字、小数点和负号,保留两位小数">
<a-input v-model="inputValue" @input="handleInput" />
</a-form-item>
</a-form>
</template>
<script>
export default {
data() {
return {
inputValue: ''
}
},
methods: {
handleInput(e) {
let value = e.target.value
// 只允许输入数字、小数点和负号
value = value.replace(/[^\d\.-]/g, '')
// 只保留两位小数
const pointIndex = value.indexOf('.')
if (pointIndex !== -1 && value.length - pointIndex > 3) {
value = value.slice(0, pointIndex + 3)
}
this.inputValue = value
}
}
}
</script>
```
在上面的代码中,我们使用正则表达式 `[^\d\.-]` 来匹配非数字、非小数点和非负号的字符,并将其替换为空字符串。然后,我们检查输入中是否包含小数点,并且小数点后面是否有超过两位数字。如果是,则将输入值截断为保留两位小数。最后,我们将处理后的值更新到 `inputValue` 变量中,以便在表单中显示。
阅读全文
相关推荐
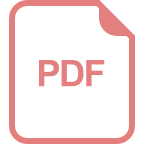













