python文档词频统计
时间: 2024-12-21 13:10:26 浏览: 10
Python 中可以使用内置的 `collections` 模块中的 `Counter` 类来进行文档词频统计。`Counter` 是一个字典子类,用于计算可迭代对象中各个元素出现的次数。以下是简单的步骤:
1. 首先,你需要读取文本文件内容,将其转换为字符串列表,或者直接对字符串进行处理。
```python
with open('yourfile.txt', 'r') as file:
text = file.read().lower() # 将所有字母转小写,便于忽略大小写差异
```
2. 使用 `split()` 或正则表达式将文本分割成单词列表。
```python
words = text.split() # 或者 words = re.findall(r'\b\w+\b', text)
```
3. 创建 `Counter` 对象并更新词频。
```python
from collections import Counter
word_counts = Counter(words)
```
4. 最后,你可以遍历 `word_counts` 来查看每个单词及其出现的次数。
```python
for word, count in word_counts.items():
print(f'{word}: {count}')
```
相关问题
利用python进行词频统计并将结果打印到另一个文档中
你可以使用Python中的collections模块来进行词频统计,具体代码如下:
```python
import collections
# 读取文本文件
with open('input.txt', 'r') as f:
text = f.read()
# 将文本分词并统计词频
words = text.split()
word_counts = collections.Counter(words)
# 将结果写入另一个文本文件
with open('output.txt', 'w') as f:
for word, count in word_counts.most_common():
f.write(f'{word}: {count}\n')
```
其中,input.txt是需要进行词频统计的文本文件,output.txt是统计结果需要写入的文本文件。你可以根据需要修改文件名和路径。
用Python对中文文档进行词频统计的代码
在Python中,我们可以使用`jieba`库来进行中文文本的分词,并结合`collections`库中的`Counter`类进行词频统计。以下是一个简单的示例:
```python
# 首先需要安装jieba库,如果未安装可以使用pip install jieba命令
import jieba
from collections import Counter
def word_frequency(text):
# 使用jieba进行中文分词
words = jieba.lcut(text)
# 使用Counter计算每个词的频率
word_counts = Counter(words)
return word_counts
# 测试示例
text = "这是一段测试的中文文档,用于演示词频统计。"
freqs = word_frequency(text)
# 输出最常出现的前10个词及其频率
for word, freq in freqs.most_common(10):
print(f"{word}: {freq}")
阅读全文
相关推荐
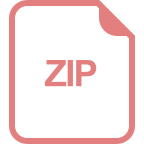
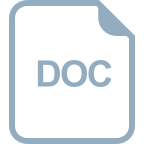
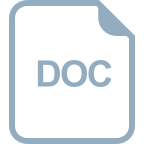
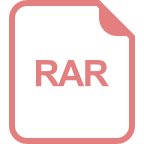
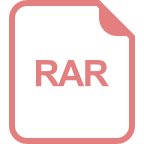
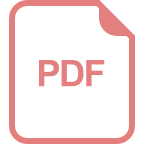
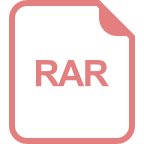
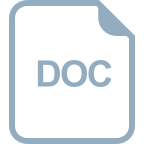
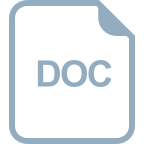





