用c语言写一个程序一个顺序栈中连续进入两个栈
时间: 2024-10-09 16:13:12 浏览: 38
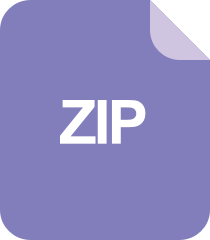
C语言 栈的实现,包含顺序栈和链栈,亲测可用

在C语言中,我们可以使用数组或链表来实现一个简单的顺序栈。如果你想在一个顺序栈中同时存储并连续操作两个栈,一种可能的做法是创建两个独立的栈结构体,并分别维护它们的顶部指针。这里是一个基本的例子,假设我们有两个栈stack1和stack2:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义栈结构体
typedef struct Stack {
int* data; // 栈数据
int top; // 当前栈顶
int capacity; // 栈容量
} Stack;
Stack* create_stack(int size) {
Stack* stack = (Stack*) malloc(sizeof(Stack));
stack->data = (int*)malloc(size * sizeof(int));
stack->top = -1;
stack->capacity = size;
return stack;
}
void push(Stack** stack, int value) {
if ((*stack)->top == (*stack)->capacity - 1) {
printf("Stack is full.\n");
return;
}
(*stack)->data[++(*stack)->top] = value;
printf("Pushed %d into stack %s.\n", value, (*stack)->top == 0 ? "stack1" : "stack2");
}
void print_stack(Stack* stack) {
for (int i = 0; i <= stack->top; ++i) {
printf("%d ", stack->data[i]);
}
printf("\n");
}
int main() {
Stack* stack1 = create_stack(5);
Stack* stack2 = create_stack(5);
// 连续操作示例
push(stack1, 1);
push(stack1, 2);
push(stack2, 3); // 现在可以将值3推入stack2
// 打印当前状态
print_stack(stack1);
print_stack(stack2);
// 示例结束,记得释放内存
free(stack1->data);
free(stack1);
free(stack2->data);
free(stack2);
return 0;
}
```
在这个例子中,当你想要向`stack2`添加元素时,只需调用`push(stack2, ...)`即可。每次`push`操作后,都会打印出哪个栈接收了新的元素。
阅读全文
相关推荐
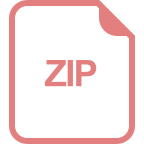
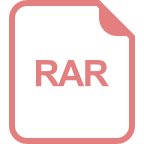


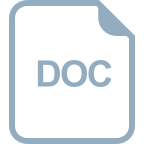
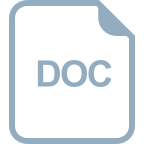
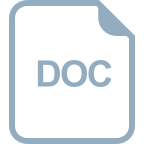
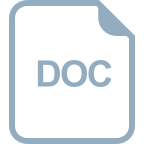
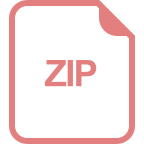
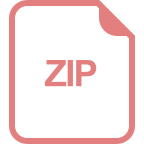
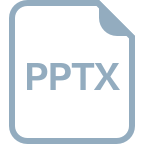
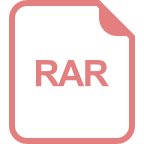
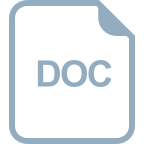
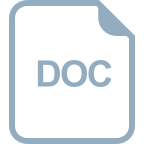
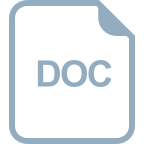
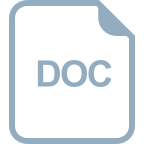
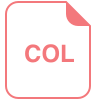