. Write a program in Java or other language to implement an email client using smtp protocol under Windows environment.
时间: 2024-10-03 08:04:16 浏览: 36
编写一个使用SMTP协议的电子邮件客户端程序,我们可以选择Java作为语言,因为它有丰富的库支持网络编程。下面是一个简单的步骤来创建这样的程序:
1. **环境设置**:
- 首先,你需要安装Java Development Kit (JDK) 和一个邮件发送库,如JavaMail API 或 Apache Commons Email。
2. **项目结构**:
- 创建一个新的Java项目,包含`EmailClient` 类负责处理SMTP交互。
3. **导入必要的库**:
```java
import javax.mail.*;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
```
4. **配置邮箱信息**:
```java
String host = "smtp.example.com";
int port = 587; // 对于SMTP通常使用TLS端口
String username = "your_email@example.com";
String password = "your_password";
```
5. **连接到SMTP服务器**:
```java
Properties properties = new Properties();
properties.put("mail.smtp.auth", "true");
properties.put("mail.smtp.starttls.enable", "true");
Session session = Session.getInstance(properties,
new Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(username, password);
}
});
Transport transport = session.getTransport("smtp");
transport.connect(host, port, username, password);
```
6. **构建并发送邮件**:
```java
MimeMessage message = new MimeMessage(session);
message.setFrom(new InternetAddress(username));
message.setTo(new InternetAddress("recipient@example.com"));
message.setSubject("Test Email");
String body = "Hello, this is a test email sent using SMTP.";
message.setText(body);
transport.sendMessage(message, message.getAllRecipients());
```
7. **关闭连接**:
```java
transport.close();
```
8. **运行和测试**:
- 编译并运行程序,检查是否能成功发送一封邮件。
```cpp
阅读全文
相关推荐
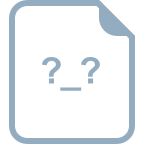
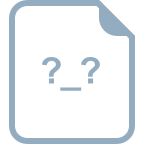
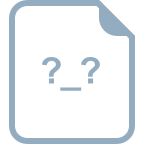
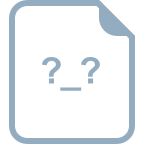
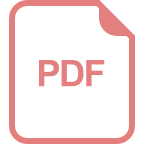
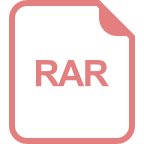
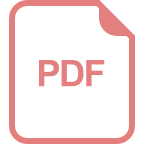
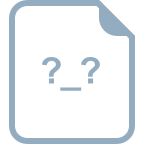
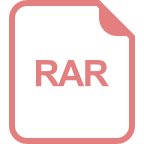
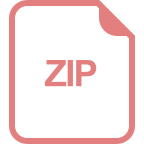
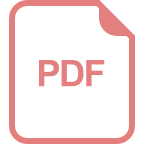
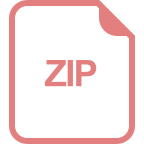
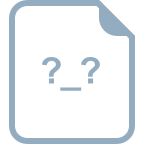
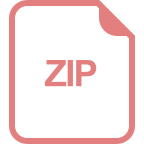
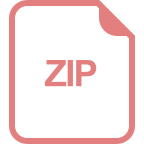
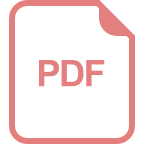
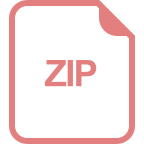