aes加密算法实现python
时间: 2024-03-26 13:33:37 浏览: 27
AES(Advanced Encryption Standard)是一种对称加密算法,常用于数据加密和解密。在Python中,可以使用`cryptography`库来实现AES加密算法。
以下是使用`cryptography`库实现AES加密算法的示例代码:
```python
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
from cryptography.hazmat.backends import default_backend
from cryptography.hazmat.primitives import padding
def aes_encrypt(key, plaintext):
# 选择AES加密算法和加密模式
cipher = Cipher(algorithms.AES(key), modes.ECB(), backend=default_backend())
encryptor = cipher.encryptor()
# 对明文进行填充
padder = padding.PKCS7(128).padder()
padded_plaintext = padder.update(plaintext) + padder.finalize()
# 加密密文
ciphertext = encryptor.update(padded_plaintext) + encryptor.finalize()
return ciphertext
def aes_decrypt(key, ciphertext):
# 选择AES解密算法和解密模式
cipher = Cipher(algorithms.AES(key), modes.ECB(), backend=default_backend())
decryptor = cipher.decryptor()
# 解密密文
padded_plaintext = decryptor.update(ciphertext) + decryptor.finalize()
# 去除填充得到明文
unpadder = padding.PKCS7(128).unpadder()
plaintext = unpadder.update(padded_plaintext) + unpadder.finalize()
return plaintext
# 测试代码
key = b'0123456789abcdef' # 密钥长度为16字节(128位)
plaintext = b'This is a secret message.'
ciphertext = aes_encrypt(key, plaintext)
decrypted_plaintext = aes_decrypt(key, ciphertext)
print("明文:", plaintext)
print("加密后的密文:", ciphertext)
print("解密后的明文:", decrypted_plaintext)
```
相关推荐
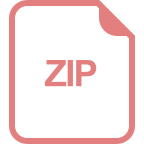











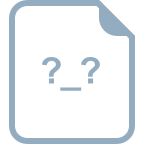
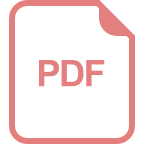
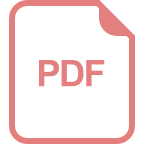