AES加密算法python实现
时间: 2023-11-10 21:05:27 浏览: 172
下面是使用Python实现AES加密的示例代码:
```python
from Crypto.Cipher import AES
import base64
# 加密函数
def encrypt(key, text):
# 将密钥进行Base64编码
key = base64.b64encode(key.encode('utf-8'))
# 将明文进行Padding,使其长度为AES块大小的整数倍
text = text + (AES.block_size - len(text) % AES.block_size) * chr(AES.block_size - len(text) % AES.block_size)
# 创建AES实例,使用CBC模式和PKCS7Padding填充方式
cipher = AES.new(key, AES.MODE_CBC, b'0000000000000000')
# 加密明文
ciphertext = cipher.encrypt(text.encode('utf-8'))
# 将密文进行Base64编码
ciphertext = base64.b64encode(ciphertext)
# 返回加密后的密文
return ciphertext.decode('utf-8')
# 解密函数
def decrypt(key, ciphertext):
# 将密钥进行Base64编码
key = base64.b64encode(key.encode('utf-8'))
# 将密文进行Base64解码
ciphertext = base64.b64decode(ciphertext)
# 创建AES实例,使用CBC模式和PKCS7Padding填充方式
cipher = AES.new(key, AES.MODE_CBC, b'0000000000000000')
# 解密密文
text = cipher.decrypt(ciphertext)
# 去除Padding
text = text[:-ord(text[-1])]
# 返回解密后的明文
return text.decode('utf-8')
# 测试代码
key = '1234567890abcdef'
text = 'Hello, AES!'
ciphertext = encrypt(key, text)
plaintext = decrypt(key, ciphertext)
print('明文:', text)
print('密文:', ciphertext)
print('解密后的明文:', plaintext)
```
需要使用 `pycryptodome` 库安装,可以通过 `pip install pycryptodome` 命令进行安装。在上面的代码中,我们使用AES算法和CBC模式进行加密和解密,并使用PKCS7Padding填充方式对明文进行Padding。在加密时,我们先对密钥进行Base64编码,然后对明文进行Padding并加密,最后将密文进行Base64编码。在解密时,我们先对密钥进行Base64编码,然后对密文进行Base64解码并解密,最后去除Padding得到明文。
阅读全文
相关推荐
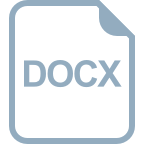
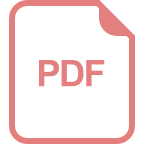










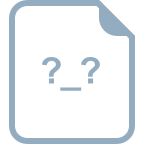
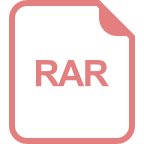