aes加密算法python实现代码
时间: 2023-07-23 14:25:39 浏览: 140
以下是Python中使用AES加密算法的示例代码:
```python
from Crypto.Cipher import AES
import base64
# 加密函数
def encrypt(text, key):
# 设定加密算法和初始向量
cipher = AES.new(key.encode('utf-8'), AES.MODE_ECB)
# 将明文进行补位操作
text = text + (AES.block_size - len(text) % AES.block_size) * chr(AES.block_size - len(text) % AES.block_size)
# 进行加密操作
ciphertext = cipher.encrypt(text.encode('utf-8'))
# 将加密结果进行base64编码
return base64.b64encode(ciphertext).decode('utf-8')
# 解密函数
def decrypt(text, key):
# 设定解密算法和初始向量
cipher = AES.new(key.encode('utf-8'), AES.MODE_ECB)
# 对密文进行base64解码
ciphertext = base64.b64decode(text)
# 进行解密操作
plaintext = cipher.decrypt(ciphertext).decode('utf-8')
# 去除补位字符
return plaintext[:-ord(plaintext[-1])]
# 测试
if __name__ == '__main__':
key = '1234567890123456' # 密钥必须是16、24或32位长
text = 'Hello, world!'
print('明文:', text)
ciphertext = encrypt(text, key)
print('加密后:', ciphertext)
plaintext = decrypt(ciphertext, key)
print('解密后:', plaintext)
```
其中,`encrypt`函数用于加密明文,`decrypt`函数用于解密密文。在此示例中,使用的加密算法为AES,加密模式为ECB,补位方式为PKCS7。请注意,密钥必须是16、24或32位长。
阅读全文
相关推荐
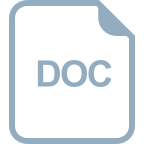
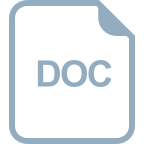
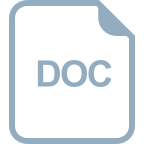










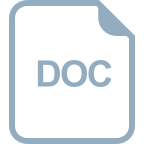
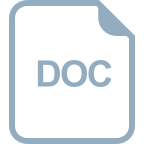
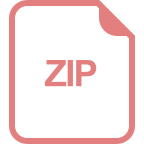
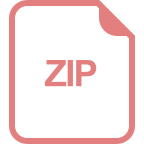
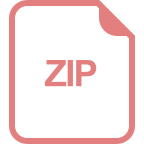