aes加密算法python代码,并详细解释
时间: 2024-10-25 09:15:16 浏览: 33
AES(Advanced Encryption Standard)是一种高级加密标准,常用于数据的安全传输。在Python中,我们可以使用`cryptography`库来实现AES加密。以下是一个简单的例子:
```python
from cryptography.hazmat.primitives.ciphers import Cipher, algorithms, modes
from cryptography.hazmat.backends import default_backend
import base64
# 密钥和初始化向量(IV)
key = b'sixteen_byte_key'
iv = b'some_iv_16_bytes'
# 加密函数
def encrypt(text, key, iv):
backend = default_backend()
cipher = Cipher(algorithms.AES(key), modes.CBC(iv), backend=backend)
encryptor = cipher.encryptor()
# 对明文进行PKCS7填充,保证加密后的块大小是16字节的倍数
padded_text = text + (16 - len(text) % 16) * chr(16 - len(text) % 16)
ciphertext = encryptor.update(padded_text.encode('utf-8')) + encryptor.finalize()
return base64.urlsafe_b64encode(ciphertext)
# 解密函数
def decrypt(ciphertext, key, iv):
backend = default_backend()
cipher = Cipher(algorithms.AES(key), modes.CBC(iv), backend=backend)
decryptor = cipher.decryptor()
decoded_ciphertext = base64.urlsafe_b64decode(ciphertext)
decrypted_text = decryptor.update(decoded_ciphertext) + decryptor.finalize().decode('utf-8')
return decrypted_text[:-ord(decrypted_text[-1])] # 去除PKCS7填充字符
# 示例
plaintext = "Hello, AES!"
encrypted_text = encrypt(plaintext, key, iv)
print("Encrypted:", encrypted_text)
decrypted_text = decrypt(encrypted_text, key, iv)
print("Decrypted:", decrypted_text)
```
在这个例子中,我们首先创建了一个AES加密器(encryptor),然后对输入的文本进行了填充和加密。加密结果会转换成Base64编码以便存储。解密时,同样使用相同的密钥和IV,从Base64解码后通过解密器还原原始文本。
阅读全文
相关推荐
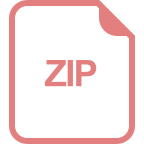
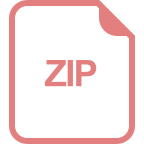










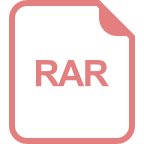
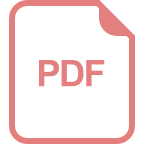
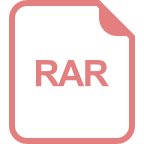
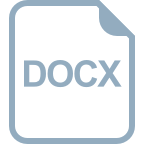
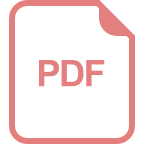