js获取当前时间加七天
时间: 2023-10-20 17:06:04 浏览: 87
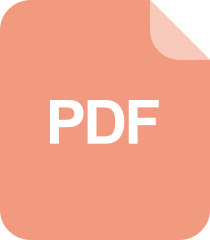
js获取当前日期前七天的方法
要在JavaScript中获取当前时间加七天,可以使用以下代码:
```javascript
// 获取当前时间
let currentDate = new Date();
// 将当前时间增加七天
currentDate.setDate(currentDate.getDate() + 7);
// 获取增加后的日期
let futureDate = currentDate.toDateString();
console.log(futureDate);
```
这段代码首先创建了一个`Date`对象,表示当前时间。然后使用`setDate()`方法将当前日期增加七天。最后,使用`toDateString()`方法将增加后的日期转换为字符串,并存储在`futureDate`变量中。你可以在控制台输出`futureDate`变量来查看结果。
阅读全文
相关推荐




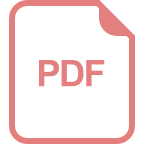









