扫雷游戏,用python代码编写
时间: 2024-12-05 12:21:42 浏览: 7
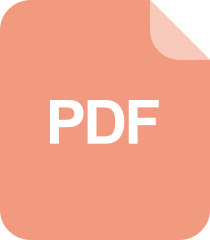
用python写扫雷游戏实例代码分享

Python 编写的扫雷游戏通常涉及基本的数据结构、用户输入处理以及游戏逻辑。这里是一个简化的版本,使用列表模拟雷区和标记状态:
```python
import random
# 定义游戏类
class Minesweeper:
def __init__(self, size=10):
self.size = size
self.board = [[0] * size for _ in range(size)]
self.mines = random.sample(range(size**2), size**2 // 10)
self.explored = set()
# 添加地雷
def place_mines(self):
for mine in self.mines:
x, y = divmod(mine, self.size)
self.board[x][y] = -1
# 显示并获取用户输入
def display(self):
print("地雷游戏")
for row in self.board:
print("|".join(str(cell) for cell in row))
try:
row, col = map(int, input("请输入行和列(例如: 3 4): ").split())
except ValueError:
return "无效输入"
# 探索邻居
def explore(self, x, y):
if (x, y) not in self.explored:
self.explored.add((x, y))
neighbors = [(x+i, y+j) for i, j in [(-1, -1), (-1, 0), (-1, 1),
(0, -1), (0, 1),
(1, -1), (1, 0), (1, 1)]]
count = sum(1 for neighbor in neighbors if neighbor in self.explored or self.board[neighbor[0]][neighbor[1]] == -1)
self.board[x][y] = count
if count == 0 and self.board[x][y] != -1:
for n in neighbors:
self.explore(n[0], n[1])
# 游戏主循环
def play(self):
self.place_mines()
while True:
self.display()
action = self.display() # 获取用户输入
if action == 'exit':
break
elif action == 'mine':
if self.board[action[0]][action[1]] == -1:
print("哎呀,你踩到地雷了!")
else:
print(f"恭喜,那是个空格!")
else:
self.explore(action[0], action[1])
if self.board[action[0]][action[1]] == 0:
print("恭喜,你找到了一个没有地雷的方块!")
# 创建并运行游戏
game = Minesweeper()
game.play()
```
这个代码实现了一个基础版的扫雷游戏,包括初始化雷区、用户输入验证、探索周围格子和游戏循环。玩家通过输入行和列来探索,遇到地雷或确认无雷区域。游戏结束后,可以选择退出或重新开始。
阅读全文
相关推荐
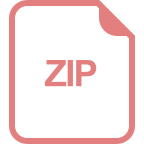
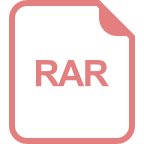












